一个文本文件记录了许多坐标信息,在C++中编写程序对文件坐标信息进行读取,并且利用数值微分函数对读取到的坐标进行直线绘制,最后利用easyx的可视化界面将绘制好的直线展示出来 要求整个代码能够成功读取坐标信息并且成功绘制
时间: 2023-12-06 18:44:54 浏览: 78
以下是一个简单的实现,其中使用了fstream库来读取文件,使用了数值微分的方法来计算直线的斜率并进行绘制,使用了EasyX库来可视化展示直线:
```c++
#include <iostream>
#include <fstream>
#include <cmath>
#include <graphics.h>
using namespace std;
// 定义坐标点的结构体
struct Point {
double x;
double y;
};
// 数值微分函数,计算两个点之间的斜率
double diff(Point p1, Point p2) {
return (p2.y - p1.y) / (p2.x - p1.x);
}
int main() {
// 打开文件
ifstream file("coords.txt");
if (!file.is_open()) {
cout << "Failed to open file!" << endl;
return 0;
}
// 读取文件中的坐标信息,并存储到数组中
const int MAX_POINTS = 1000;
Point points[MAX_POINTS];
int count = 0;
while (!file.eof()) {
// 读取一行数据
string line;
getline(file, line);
if (line.empty()) {
continue;
}
// 解析坐标点
double x, y;
sscanf(line.c_str(), "%lf,%lf", &x, &y);
points[count].x = x;
points[count].y = y;
count++;
}
// 初始化EasyX图形界面
initgraph(800, 600);
// 绘制直线
setlinestyle(PS_SOLID, 2); // 设置线条样式
setlinecolor(RGB(255, 0, 0)); // 设置线条颜色
for (int i = 0; i < count - 1; i++) {
double k = diff(points[i], points[i + 1]); // 计算斜率
double b = points[i].y - k * points[i].x; // 计算截距
line(points[i].x, points[i].y, points[i + 1].x, points[i + 1].y); // 绘制直线
}
// 关闭EasyX图形界面
getch();
closegraph();
return 0;
}
```
需要注意的是,以上代码中的 `coords.txt` 文件应该与可执行文件放在同一目录下,文件格式为每行一个坐标点,格式为 `x,y`,例如:
```
1.2,3.4
5.6,7.8
9.0,1.2
...
```
阅读全文
相关推荐

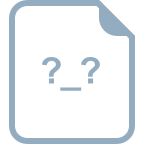
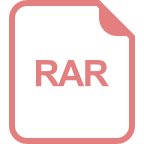
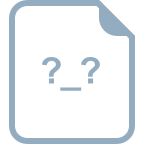
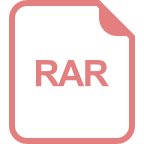
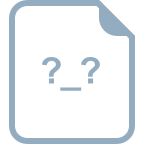
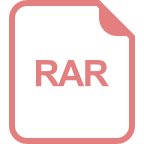
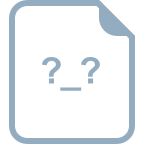
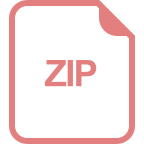
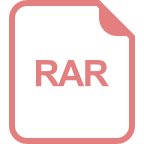
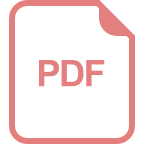
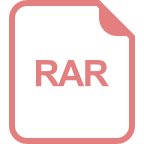
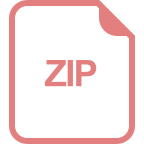
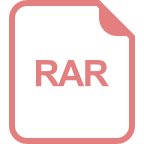
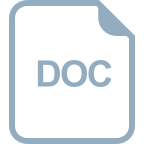
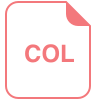
