完成 MyString 类的实现,使程序正确运行 #include <iostream> #include <cstring> using namespace std; class MyString { public: // 无参构造函数 MyString(); // 构造函数,传入一个C语言风格字符串 MyString(const char *s); // 拷贝构造函数 MyString(const MyString &s); // 析构函数 ~MyString(); // 将C语言风格的字符串赋值给MyString对象 MyString& operator=(const char *s); // 将一个MyString对象赋值给另一个MyString对象 MyString& operator=(const MyString &s); // 将这个MyString对象与C语言风格的字符串相连 MyString& operator+=(const char *s); // 将这个MyString对象与另一个MyString对象相连 MyString& operator+=(const MyString &s); // 返回下标为pos的字符 char& operator[](int pos); // 返回子串 [pos, pos+count) // 若请求的子串越过字符串的结尾,即count大于size() - pos,则返回的子串为[pos, size()) // 若pos不在字符串的下标范围内,返回空的MyString对象 // 若count == -1,返回子串[pos, size()) MyString substr(int pos = 0, int count = -1); // 字符串的长度 int size() const; // 返回C语言风格的字符串 const char* c_str() const; private: char *m_buf; int m_size; }; // 请在此处填写 int main() { char s[101]; cin.getline(s, 101); int pos, count; // 创建、拷贝、赋值 MyString s1(s), s2(s1), s3, s4, s5; s3 = s; s4 = s1; cout << "s1: " << s1.c_str() << endl; cout << "s2: " << s2.c_str() << endl; cout << "s3: " << s3.c_str() << endl; cout << "s4: " << s4.c_str() << endl; // 拼接 s3 += s; s4 += s1; cout << "s3 += s: " << s3.c_str() << endl; cout << "s4 += s1: " << s4.c_str() << endl; // 下标 cin >> pos; s4[pos] = 'm'; cout << "s4[pos] = 'm': " << s4.c_str() << endl; // 取子串 cin >> pos >> count; s5 = s4.substr(pos, count); cout << "s5: " << s5.c_str() << endl; // 以后还可以重载<<直接输出一个MyString对象哦 TODO return 0; }
时间: 2024-03-23 18:40:46 浏览: 133
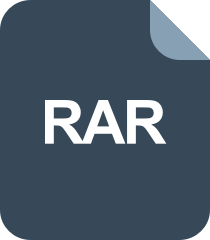
MyString类(很全并且编译通过)
实现如下:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
public:
// 无参构造函数
MyString() : m_buf(nullptr), m_size(0) {}
// 构造函数,传入一个C语言风格字符串
MyString(const char *s) : m_buf(nullptr), m_size(0) {
if (s != nullptr) {
m_size = strlen(s);
m_buf = new char[m_size + 1];
strcpy(m_buf, s);
}
}
// 拷贝构造函数
MyString(const MyString &s) : m_buf(nullptr), m_size(s.m_size) {
if (m_size > 0) {
m_buf = new char[m_size + 1];
strcpy(m_buf, s.m_buf);
}
}
// 析构函数
~MyString() {
delete[] m_buf;
}
// 将C语言风格的字符串赋值给MyString对象
MyString& operator=(const char *s) {
if (s == nullptr) {
delete[] m_buf;
m_buf = nullptr;
m_size = 0;
}
else {
int len = strlen(s);
if (len > m_size) {
delete[] m_buf;
m_buf = new char[len + 1];
m_size = len;
}
strcpy(m_buf, s);
}
return *this;
}
// 将一个MyString对象赋值给另一个MyString对象
MyString& operator=(const MyString &s) {
if (this != &s) {
if (s.m_buf == nullptr) {
delete[] m_buf;
m_buf = nullptr;
m_size = 0;
}
else {
int len = s.m_size;
if (len > m_size) {
delete[] m_buf;
m_buf = new char[len + 1];
m_size = len;
}
strcpy(m_buf, s.m_buf);
}
}
return *this;
}
// 将这个MyString对象与C语言风格的字符串相连
MyString& operator+=(const char *s) {
int len = strlen(s);
char *new_buf = new char[m_size + len + 1];
if (m_buf != nullptr) {
strcpy(new_buf, m_buf);
delete[] m_buf;
}
strcpy(new_buf + m_size, s);
m_buf = new_buf;
m_size += len;
return *this;
}
// 将这个MyString对象与另一个MyString对象相连
MyString& operator+=(const MyString &s) {
return (*this += s.c_str());
}
// 返回下标为pos的字符
char& operator[](int pos) {
return m_buf[pos];
}
// 返回子串 [pos, pos+count)
// 若请求的子串越过字符串的结尾,即count大于size() - pos,则返回的子串为[pos, size())
// 若pos不在字符串的下标范围内,返回空的MyString对象
// 若count == -1,返回子串[pos, size())
MyString substr(int pos = 0, int count = -1) {
if (pos < 0 || pos >= m_size) {
return MyString();
}
if (count == -1) {
count = m_size - pos;
}
else if (count > m_size - pos) {
count = m_size - pos;
}
char *new_buf = new char[count + 1];
strncpy(new_buf, m_buf + pos, count);
new_buf[count] = '\0';
MyString res(new_buf);
delete[] new_buf;
return res;
}
// 字符串的长度
int size() const {
return m_size;
}
// 返回C语言风格的字符串
const char* c_str() const {
return m_buf;
}
private:
char *m_buf;
int m_size;
};
int main() {
char s[101];
cin.getline(s, 101);
int pos, count;
// 创建、拷贝、赋值
MyString s1(s), s2(s1), s3, s4, s5;
s3 = s;
s4 = s1;
cout << "s1: " << s1.c_str() << endl;
cout << "s2: " << s2.c_str() << endl;
cout << "s3: " << s3.c_str() << endl;
cout << "s4: " << s4.c_str() << endl;
// 拼接
s3 += s;
s4 += s1;
cout << "s3 += s: " << s3.c_str() << endl;
cout << "s4 += s1: " << s4.c_str() << endl;
// 下标
cin >> pos;
s4[pos] = 'm';
cout << "s4[pos] = 'm': " << s4.c_str() << endl;
// 取子串
cin >> pos >> count;
s5 = s4.substr(pos, count);
cout << "s5: " << s5.c_str() << endl;
return 0;
}
```
阅读全文
相关推荐
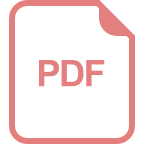











