描述 请重载复数类的流插入和流提取运算符,实现复数类的输出和输入。 #include<iostream> #include <string> using namespace std; //--------------------- class Complex { private: double real,imag; public: Complex(){ real=imag=0; } Complex(double r){ real=r;imag=0; } Complex(double r,double i){ real=r;imag=i; } // 在此处补充你的代码 int main() { Complex c; cin>>c; cout<<c; } 输入 实部和虚部以英文逗号分隔 输出 实部+虚部j
时间: 2024-01-07 22:04:42 浏览: 33
完整的复数类的代码如下:
```c++
#include<iostream>
#include <string>
using namespace std;
class Complex {
private:
double real, imag;
public:
Complex() {
real = imag = 0;
}
Complex(double r) {
real = r;
imag = 0;
}
Complex(double r, double i) {
real = r;
imag = i;
}
friend ostream& operator<<(ostream& os, const Complex& c); // 友元函数声明,重载输出运算符
friend istream& operator>>(istream& is, Complex& c); // 友元函数声明,重载输入运算符
};
ostream& operator<<(ostream& os, const Complex& c) { // 重载输出运算符
os << c.real;
if (c.imag >= 0) {
os << "+";
}
os << c.imag << "j";
return os;
}
istream& operator>>(istream& is, Complex& c) { // 重载输入运算符
string input;
char comma;
is >> input; // 输入格式为 "实部,虚部"
int pos = input.find(",");
if (pos == string::npos) { // 找不到逗号,输入不合法
is.setstate(ios::failbit); // 设置失败状态位
return is;
}
try {
c.real = stod(input.substr(0, pos)); // 将字符串转换为 double 类型
c.imag = stod(input.substr(pos + 1));
}
catch (...) { // 转换失败,输入不合法
is.setstate(ios::failbit); // 设置失败状态位
}
return is;
}
int main() {
Complex c;
if (cin >> c) {
cout << c;
}
else {
cout << "输入不合法!" << endl;
}
return 0;
}
```
其中,友元函数 `operator<<` 和 `operator>>` 分别用于重载输出运算符和输入运算符。在 `operator<<` 中,我们首先输出实部,然后判断虚部的符号,最后输出虚部和 "j"。在 `operator>>` 中,我们首先读入一个字符串,然后找到逗号的位置,将逗号前后的字符串转换为 double 类型,最后赋值给实部和虚部。如果输入不合法(例如缺少逗号或无法将字符串转换为 double 类型),我们设置输入流的失败状态位,以便在主函数中进行错误处理。
相关推荐
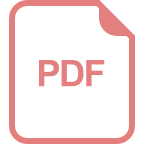
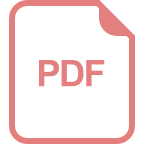
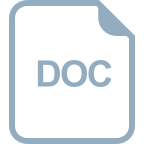














