C++算术表达式求值编程
时间: 2023-08-30 11:05:12 浏览: 86
C++中可以使用栈来实现算术表达式求值。具体步骤如下:
1. 定义一个栈,用来存储数字和运算符。
2. 遍历表达式中的每个字符,对于每个字符做如下处理:
- 如果是数字,将其压入栈中。
- 如果是运算符,从栈中弹出两个数字进行运算,并将结果压入栈中。
3. 遍历完整个表达式后,栈中只剩下一个元素,即为表达式的值。
下面是一份示例代码:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <cctype>
using namespace std;
int evaluate(string expr) {
stack<int> s; // 定义一个栈
int num = 0;
for (char c : expr) {
if (isdigit(c)) { // 如果是数字
num = num * 10 + (c - '0'); // 构造数字
} else if (c == '+' || c == '-' || c == '*' || c == '/') { // 如果是运算符
s.push(num); // 将数字压入栈中
num = 0;
int op1 = s.top(); s.pop();
int op2 = s.top(); s.pop();
switch (c) {
case '+': s.push(op2 + op1); break;
case '-': s.push(op2 - op1); break;
case '*': s.push(op2 * op1); break;
case '/': s.push(op2 / op1); break;
}
}
}
s.push(num); // 将最后一个数字压入栈中
int result = s.top(); s.pop();
return result;
}
int main() {
string expr = "1+2*3-4/2";
int result = evaluate(expr);
cout << result << endl; // 输出:5
return 0;
}
```
在上面的代码中,我们遍历表达式中的每个字符,如果是数字就将其构造成一个整数,如果是运算符就从栈中弹出两个数字进行运算,并将结果压入栈中。遍历完整个表达式后,栈中只剩下一个元素,即为表达式的值。
阅读全文
相关推荐
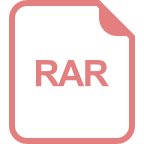
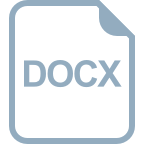
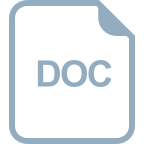















