python将基于相机坐标系获得的3D坐标点转换为世界坐标系下的3D坐标点
时间: 2023-09-17 20:10:04 浏览: 108
要将基于相机坐标系获得的3D坐标点转换为世界坐标系下的3D坐标点,你需要知道相机的内参和外参。相机的内参包括焦距、光心等参数,而外参包括相机在世界坐标系下的位置和朝向。
以下是一个简单的示例代码,使用OpenCV库来进行相机坐标系到世界坐标系的转换:
```python
import numpy as np
import cv2
# 相机内参
fx = 500 # x轴方向的焦距
fy = 500 # y轴方向的焦距
cx = 320 # x轴方向的光心位置
cy = 240 # y轴方向的光心位置
# 相机外参
camera_position = np.array([0, 0, 0]) # 相机在世界坐标系下的位置
camera_orientation = np.array([0, 0, 0]) # 相机在世界坐标系下的朝向
# 基于相机坐标系获得的3D点
camera_point = np.array([100, 200, 300])
# 构建相机投影矩阵
camera_matrix = np.array([[fx, 0, cx],
[0, fy, cy],
[0, 0, 1]])
# 构建旋转矩阵
rotation_matrix = cv2.Rodrigues(camera_orientation)[0]
# 构建平移矩阵
translation_matrix = -np.dot(rotation_matrix, camera_position)
# 将相机坐标系下的点转换为齐次坐标
camera_point_homogeneous = np.append(camera_point, 1)
# 相机坐标系到世界坐标系的转换
world_point_homogeneous = np.dot(np.dot(rotation_matrix, np.linalg.inv(camera_matrix)), camera_point_homogeneous) + translation_matrix
# 转换为非齐次坐标
world_point = world_point_homogeneous[:3] / world_point_homogeneous[3]
print("World Point:", world_point)
```
请注意,这只是一个简单的示例,实际应用中可能需要更复杂的计算和参数设置。另外,你需要确保相机内参和外参的准确性,以获得准确的转换结果。
阅读全文
相关推荐
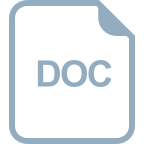
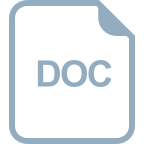
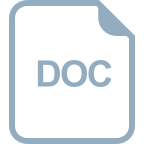













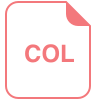

