pygame侦测鼠标坐标
时间: 2023-07-27 20:06:21 浏览: 157
要侦测鼠标坐标,可以使用pygame.mouse.get_pos()函数。这个函数返回一个元组,其中包含当前鼠标的x和y坐标,例如:
```
import pygame
pygame.init()
# 创建窗口
screen = pygame.display.set_mode((640, 480))
# 游戏循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 获取鼠标坐标
mouse_pos = pygame.mouse.get_pos()
# 在窗口上绘制鼠标坐标
screen.fill((0, 0, 0))
font = pygame.font.Font(None, 36)
text = font.render("Mouse position: {}".format(mouse_pos), True, (255, 255, 255))
screen.blit(text, (10, 10))
pygame.display.update()
```
这个示例代码会创建一个窗口,并在窗口上显示当前鼠标的坐标。当鼠标移动时,坐标也会随之改变。
相关问题
pygame 鼠标坐标
Pygame是一个流行的Python库,用于创建2D游戏和图形应用程序。在游戏中,鼠标坐标是非常重要的,它们可以帮助你跟踪用户的交互。当你使用Pygame获取鼠标坐标时,通常会通过`pygame.mouse.get_pos()`函数来实现。这个函数返回一个元组,包含鼠标的当前x和y位置,这两个值都是相对于屏幕左上角的像素。
例如:
```python
import pygame
# 初始化Pygame
pygame.init()
# 设置窗口大小
screen = pygame.display.set_mode((800, 600))
while True:
# 检查事件
for event in pygame.event.get():
if event.type == pygame.MOUSEMOTION:
mouse_pos = pygame.mouse.get_pos()
x, y = mouse_pos # 分离出x和y坐标
print(f"鼠标位置: ({x}, {y})")
# 更新屏幕
pygame.display.update()
```
在这个例子中,每当鼠标移动时,程序就会打印出新的鼠标坐标。
pygame如何获取鼠标坐标
在Pygame中,你可以使用`pygame.mouse.get_pos()`函数来获取当前鼠标的标。这个函数会返回一个包含鼠标x和y坐标的元组。你可以将这个函数放在游戏的主循环中,以便实时获取鼠标的位置。
下面是一个简单的示例代码,演示了如何使用Pygame获取鼠标坐标:
```python
import pygame
import sys
pygame.init()
# 设置窗口大小
screen = pygame.display.set_mode((800, 600))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 获取鼠标坐标
mouse_pos = pygame.mouse.get_pos()
x, y = mouse_pos
# 在窗口上绘制鼠标坐标
screen.fill((255, 255, 255))
font = pygame.font.Font(None, 36)
text = font.render(f"Mouse Position: ({x}, {y})", True, (0, 0, 0))
screen.blit(text, (10, 10))
pygame.display.flip()
```
这段代码创建了一个800x600的窗口,并在窗口上实时显示鼠标的坐标。当你运行这段代码时,你会看到一个窗口,其中显示了当前鼠标的x和y坐标。
希望这个示例对你有帮助!
阅读全文
相关推荐
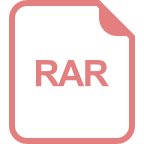
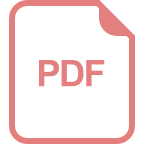
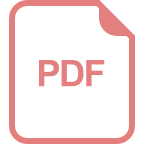













