二叉排序树建立与查找
时间: 2023-08-21 09:22:02 浏览: 69
二叉排序树的建立已经在上一个问题中介绍过了,这里给出二叉排序树的查找操作。
二叉排序树的查找操作也很简单,基本思路就是从根节点开始,逐个比较节点的值,直到找到目标节点或者遍历到叶子节点为止。
代码实现如下:
```c++
// 在二叉排序树中查找指定值
TreeNode* searchBST(TreeNode* root, int val) {
if (!root) return NULL;
if (root->val == val) return root;
if (val < root->val) {
return searchBST(root->left, val);
} else {
return searchBST(root->right, val);
}
}
```
其中,searchBST 函数接收一个二叉排序树的根节点 root 和一个目标值 val,返回值为指向目标节点的指针。如果找不到目标节点,返回 NULL。
需要注意的是,这里的查找操作是基于二叉排序树的性质进行的,因此时间复杂度为 O(log n),其中 n 为节点个数。当二叉排序树退化为链表时,查找操作最坏情况下会达到 O(n) 的时间复杂度。因此,在实际应用中,我们需要对二叉排序树进行平衡处理,以保证其效率。
相关问题
二叉排序树的建立与查找
建立二叉排序树的过程可以通过以下步骤实现:
1. 创建一个空树作为二叉排序树的根节点。
2. 从待插入元素中选择一个作为根节点。
3. 遍历待插入元素,依次将每一个元素插入到树中。
4. 对于每一个待插入的元素,从根节点开始比较,如果该元素小于当前节点的值,则将其插入到当前节点的左子树中,否则将其插入到当前节点的右子树中。
二叉排序树的查找可以通过以下步骤实现:
1. 从根节点开始比较,如果待查找元素等于当前节点的值,则返回当前节点。
2. 如果待查找元素小于当前节点的值,则在当前节点的左子树中递归查找。
3. 如果待查找元素大于当前节点的值,则在当前节点的右子树中递归查找。
4. 如果查找到叶子节点仍然没有找到待查找元素,则说明该元素不存在于二叉排序树中。
二叉排序树的建立、查找、二分法查找
1. 二叉排序树的建立:
二叉排序树(Binary Search Tree)是一种特殊的二叉树,其左子树上的所有节点的值都小于根节点的值,右子树上的所有节点的值都大于根节点的值。因此,二叉排序树的中序遍历得到的序列是有序的。
建立二叉排序树的方法是,从根节点开始,依次将新插入的节点与当前节点的值作比较,如果小于当前节点,则以当前节点的左子节点为根节点继续比较;如果大于当前节点,则以当前节点的右子节点为根节点继续比较,直到找到一个空的位置插入新节点。
2. 二叉排序树的查找:
查找二叉排序树中的一个节点,可以从根节点开始依次比较节点的值,如果要查找的值小于当前节点的值,则以当前节点的左子节点为根节点继续比较;如果要查找的值大于当前节点的值,则以当前节点的右子节点为根节点继续比较。如果找到了要查找的值,则返回该节点,否则返回空。
3. 二分法查找:
二分法查找是一种在有序数组中查找特定元素的算法。其基本思想是,将数组分成两个部分,判断要查找的元素在哪个部分中,然后在该部分中继续查找。
具体实现是,定义两个指针,一个指向数组的第一个元素,一个指向数组的最后一个元素。每次将中间位置的元素与要查找的元素比较,如果相等,则返回该元素的下标;如果中间位置的元素大于要查找的元素,则在左半部分继续查找;如果中间位置的元素小于要查找的元素,则在右半部分继续查找。重复以上过程,直到找到要查找的元素或者确定该元素不存在为止。
相关推荐
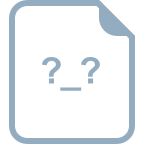
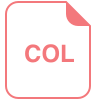











