def reset(self, screen, robot, new_robot, dusts, agent): self.screen = screen self.robot = robot self.new_robot = new_robot self.dusts = dusts self.current_dusts = len(self.dusts) nearest_dust, self.distance = self.get_nearest_dust() self.agent = agent robot_x, robot_y = self.robot.rect.centerx, self.robot.rect.centery dust_x, dust_y = nearest_dust.rect.centerx, nearest_dust.rect.centery sin = (robot_x - dust_x) / self.distance cos = (robot_y - dust_y) / self.distance dust_angle = math.atan2(sin, cos) / math.pi * 180 return self.robot.rect.centerx, self.robot.rect.centery, self.robot.angle, nearest_dust.rect.centerx, nearest_dust.rect.centery, dust_angle, self.distance
时间: 2024-04-09 16:31:38 浏览: 15
这是Environment类中的一个方法reset,它接受一些来初始化环境的状态,并返回初始状态的。
在方法内部,传入的参数screen、robot、new_robot、dusts和agent会被分别赋值给对应的属性。
接下来,通过调用get_nearest_dust方法来获取最近的尘埃物体,并将结果赋值给nearest_dust和distance属性。
然后,机器人和最近尘埃物体的位置信息会被获取,并计算机器人与尘埃之间的角度。这些信息会被用来表示初始状态。
最后,方法返回机器人和尘埃的位置信息、机器人的角度、尘埃的位置信息、尘埃与机器人之间的角度和距离。
还有其他问题吗?
相关问题
class Environment: def __init__(self): self.screen = None self.robot = None self.new_robot = None self.dusts = None self.current_dusts = None self.nearest_dust, self.distance = None, None self.agent = None
这是一个名为Environment的类,它具有一些属性和方法用于描述一个环境。属性包括screen(屏幕)、robot(机器人)、new_robot(新机器人)、dusts(尘埃)、current_dusts(当前尘埃)、nearest_dust(最近的尘埃)和distance(距离),以及agent(代理)。
你的第一个问题是什么?
def __init__(self, node_type_list, standardization, scenes=None, attention_radius=None, robot_type=None): self.scenes = scenes self.node_type_list = node_type_list self.attention_radius = attention_radius self.NodeType = NodeTypeEnum(node_type_list) self.robot_type = robot_type self.standardization = standardization self.standardize_param_memo = dict() self._scenes_resample_prop = None
这是一个 Python 代码的类的初始化函数 `__init__`。它接收参数:
- node_type_list:节点类型列表
- standardization:标准化参数
- scenes:场景(可选)
- attention_radius:关注半径(可选)
- robot_type:机器人类型(可选)
在函数内部,它会定义和初始化多个类的成员变量:
- self.scenes:场景
- self.node_type_list:节点类型列表
- self.attention_radius:关注半径
- self.NodeType:节点类型枚举
- self.robot_type:机器人类型
- self.standardization:标准化参数
- self.standardize_param_memo:标准化参数备忘录,一个字典类型
- self._scenes_resample_prop:场景重采样比例,初始值为 None。
相关推荐
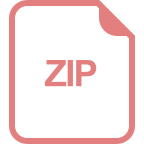












