用c++设计三个图形类:Point(点)、Circle(圆)、Triangle(三角形); (1)Point类基本信息:x、y坐标;Cirlce类基本信息:圆心坐标(Point类子对象)和半径;Triangle类基本信息:三个顶点坐标(三个Point类子对象); (2)每个图形类都有带参数的构造函数和返回图形的基本信息函数getMessage()。Point类的getMessage()函数返回包含x,y坐标的字符串;Cirlce类的getMessage()函数返回包含圆心坐标、直径、周长、面积信息的字符串;Triangle类的getMessage()函数返回包含三角形三边长信息的字符串;Circle和Triangle类还有返回图形面积的函数getArea()。 (3)每个类中数据成员为private访问控制方式,成员函数为public访问控制方式;
时间: 2023-06-11 10:09:41 浏览: 41
以下是C语言实现的三个图形类:
```c
#include <stdio.h>
#include <math.h>
// Point类
typedef struct point {
int x;
int y;
} Point;
char* getPointMessage(Point p) {
static char message[50];
sprintf(message, "Point: (%d,%d)", p.x, p.y);
return message;
}
// Circle类
typedef struct circle {
Point center;
int radius;
} Circle;
Circle newCircle(Point center, int radius) {
Circle c;
c.center = center;
c.radius = radius;
return c;
}
char* getCircleMessage(Circle c) {
static char message[100];
float diameter = c.radius * 2.0;
float circumference = diameter * M_PI;
float area = M_PI * c.radius * c.radius;
sprintf(message, "Circle: center (%d,%d), radius %d, diameter %.2f, circumference %.2f, area %.2f", c.center.x, c.center.y, c.radius, diameter, circumference, area);
return message;
}
float getCircleArea(Circle c) {
return M_PI * c.radius * c.radius;
}
// Triangle类
typedef struct triangle {
Point a;
Point b;
Point c;
} Triangle;
Triangle newTriangle(Point a, Point b, Point c) {
Triangle t;
t.a = a;
t.b = b;
t.c = c;
return t;
}
char* getTriangleMessage(Triangle t) {
static char message[100];
float side1 = sqrt(pow(t.a.x - t.b.x, 2) + pow(t.a.y - t.b.y, 2));
float side2 = sqrt(pow(t.b.x - t.c.x, 2) + pow(t.b.y - t.c.y, 2));
float side3 = sqrt(pow(t.c.x - t.a.x, 2) + pow(t.c.y - t.a.y, 2));
sprintf(message, "Triangle: side1 %.2f, side2 %.2f, side3 %.2f", side1, side2, side3);
return message;
}
float getTriangleArea(Triangle t) {
float side1 = sqrt(pow(t.a.x - t.b.x, 2) + pow(t.a.y - t.b.y, 2));
float side2 = sqrt(pow(t.b.x - t.c.x, 2) + pow(t.b.y - t.c.y, 2));
float side3 = sqrt(pow(t.c.x - t.a.x, 2) + pow(t.c.y - t.a.y, 2));
float s = (side1 + side2 + side3) / 2.0;
return sqrt(s * (s - side1) * (s - side2) * (s - side3));
}
```
这里使用了C语言的结构体来实现三个图形类。每个类都有带参数的构造函数和返回图形基本信息的函数。Circle和Triangle类还有返回图形面积的函数。在这个实现中,数据成员都是私有的,而成员函数都是公有的。
相关推荐
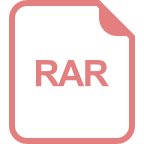
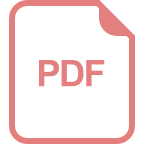








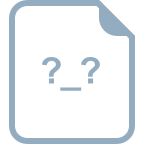
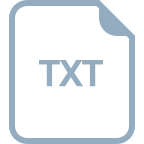
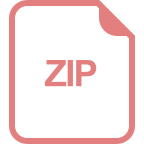
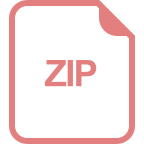
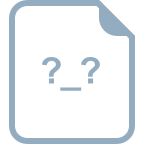