用python把下面所有步骤写成代码,用re,argparse,pandas。可以实现通过命令把txt文件的指定内容另存为CSV,生成的CSV文件自动命名并保存到当前文件夹。 弹出指定标题的曲线图 1.读取txt文件 2.遍历文档,先找到关键字ABCDE所在行数,然后保存从这一行到末尾的所有内容,删除重复内容的行,没有Header,另存为到到CSV。 3.将CSV中的文本内容,以空格为分隔符,进行分列 4.插入已经设置好的标题 5.根据标题和数据,生成曲线图。
时间: 2023-07-14 17:12:44 浏览: 50
以下是代码实现:
```python
import re
import argparse
import pandas as pd
import matplotlib.pyplot as plt
# 命令行参数解析
parser = argparse.ArgumentParser(description='Process specified content in a text file and save it as CSV.')
parser.add_argument('filename', type=str, help='the name of the input text file')
parser.add_argument('keyword', type=str, help='the keyword to search for in the text file')
parser.add_argument('title', type=str, help='the title of the generated plot')
args = parser.parse_args()
# 读取txt文件
with open(args.filename, 'r') as f:
file_content = f.read()
# 找到关键字所在的行数
lines = file_content.split('\n')
start_line_idx = None
for i, line in enumerate(lines):
if args.keyword in line:
start_line_idx = i
break
# 保存从关键字所在行到末尾的所有内容
content = '\n'.join(lines[start_line_idx:])
# 删除重复内容的行
unique_lines = list(set(content.split('\n')))
clean_content = '\n'.join(unique_lines)
# 另存为CSV
output_file = args.keyword + '.csv'
pd.DataFrame(clean_content.split('\n')).to_csv(output_file, index=False, header=False)
# 读取CSV文件并进行分列
df = pd.read_csv(output_file, sep=' ', header=None)
df.columns = ['col1', 'col2', 'col3', 'col4', 'col5']
# 插入标题
df.insert(0, 'title', [args.title] * len(df))
# 生成曲线图
plt.plot(df['col1'], df['col2'], label='col2')
plt.plot(df['col1'], df['col3'], label='col3')
plt.plot(df['col1'], df['col4'], label='col4')
plt.plot(df['col1'], df['col5'], label='col5')
plt.title(args.title)
plt.legend()
plt.show()
```
以上代码中,我们使用了Python内置的argparse模块来解析命令行参数,使用了正则表达式和Pandas库来处理文本文件,使用了Matplotlib库来生成曲线图。通过在命令行中输入相应的参数,即可实现指定内容的另存为CSV,并生成相应的曲线图。
相关推荐
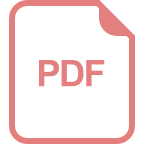
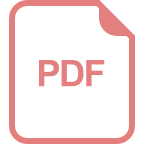
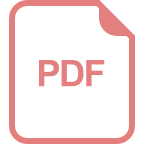
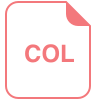
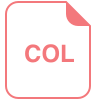
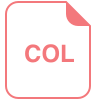
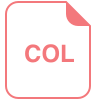









