用c语言实现给你一批入栈序列,请输出他们的出栈序列。
时间: 2023-04-12 11:00:29 浏览: 96
可以使用栈来实现,具体步骤如下:
1. 定义一个栈,用来存储入栈序列中的元素。
2. 遍历入栈序列,对于每一个元素,将其入栈。
3. 每次入栈后,检查栈顶元素是否与出栈序列的下一个元素相同,如果相同,则将栈顶元素出栈,并将出栈序列的指针向后移动一位。
4. 重复步骤3,直到栈为空或者栈顶元素与出栈序列的下一个元素不相同。
5. 如果遍历完入栈序列后,栈为空,则说明入栈序列可以按照出栈序列的顺序出栈;否则,说明无法按照出栈序列的顺序出栈。
下面是用C语言实现的代码:
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, int x) {
if (is_full(s)) {
printf("Stack is full\n");
exit(1);
}
s->data[++s->top] = x;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty\n");
exit(1);
}
return s->data[s->top--];
}
int peek(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty\n");
exit(1);
}
return s->data[s->top];
}
void print_stack(Stack *s) {
int i;
for (i = s->top; i >= 0; i--) {
printf("%d ", s->data[i]);
}
printf("\n");
}
void check(Stack *s, int *out, int n) {
int i = 0, j = 0;
while (i < n) {
if (!is_empty(s) && peek(s) == out[j]) {
pop(s);
j++;
} else {
push(s, i++);
}
}
while (!is_empty(s) && peek(s) == out[j]) {
pop(s);
j++;
}
if (is_empty(s)) {
printf("The input sequence can be converted to the output sequence\n");
} else {
printf("The input sequence cannot be converted to the output sequence\n");
}
}
int main() {
Stack s;
int input[] = {1, 2, 3, 4, 5};
int output[] = {4, 5, 3, 2, 1};
int n = sizeof(input) / sizeof(int);
init(&s);
check(&s, output, n);
return 0;
}
阅读全文
相关推荐
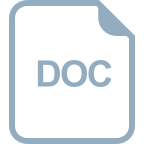
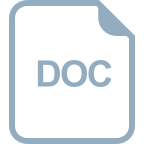
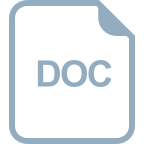


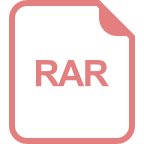







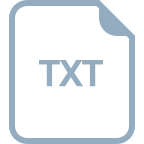
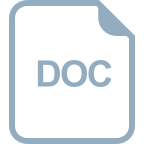
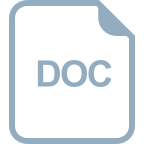

