用C++语言构建无向网,并在此基础上求得指定起点和终点的最短路径,并且添加中文注释
时间: 2024-06-14 09:05:26 浏览: 87
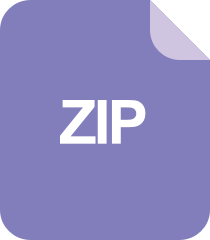
一个用C++写的求最短路径的代码

以下是使用C++语言构建无向网并求得指定起点和终点的最短路径的示例代码,同时添加了中文注释:
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <climits>
using namespace std;
// 定义无向网的边结构体
struct Edge {
int dest; // 目标顶点
int weight; // 边的权重
};
// 构建无向网的类
class Graph {
private:
int V; // 顶点的数量
vector<vector<Edge>> adj; // 邻接表表示的图
public:
Graph(int v) {
V = v;
adj.resize(V);
}
// 添加边
void addEdge(int src, int dest, int weight) {
Edge edge;
edge.dest = dest;
edge.weight = weight;
adj[src].push_back(edge);
// 由于是无向网,所以需要添加反向边
edge.dest = src;
adj[dest].push_back(edge);
}
// 求最短路径
vector<int> shortestPath(int src, int dest) {
vector<int> dist(V, INT_MAX); // 存储起点到各个顶点的最短距离
vector<int> prev(V, -1); // 存储最短路径上每个顶点的前驱顶点
vector<bool> visited(V, false); // 记录顶点是否已访问
// 使用优先队列实现Dijkstra算法
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
pq.push(make_pair(0, src));
dist[src] = 0;
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
visited[u] = true;
// 遍历与当前顶点相邻的顶点
for (const auto& edge : adj[u]) {
int v = edge.dest;
int weight = edge.weight;
if (!visited[v] && dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
prev[v] = u;
pq.push(make_pair(dist[v], v));
}
}
}
// 构建最短路径
vector<int> path;
int current = dest;
while (current != -1) {
path.push_back(current);
current = prev[current];
}
reverse(path.begin(), path.end());
return path;
}
};
int main() {
// 创建一个包含6个顶点的无向网
Graph graph(6);
graph.addEdge(0, 1, 2);
graph.addEdge(0, 2, 4);
graph.addEdge(1, 2, 1);
graph.addEdge(1, 3, 4);
graph.addEdge(2, 3, 1);
graph.addEdge(2, 4, 3);
graph.addEdge(3, 4, 1);
graph.addEdge(3, 5, 5);
graph.addEdge(4, 5, 2);
// 求从顶点0到顶点5的最短路径
vector<int> shortestPath = graph.shortestPath(0, 5);
// 输出最短路径
cout << "最短路径:";
for (int i = 0; i < shortestPath.size(); i++) {
cout << shortestPath[i];
if (i != shortestPath.size() - 1) {
cout << " -> ";
}
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
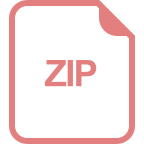




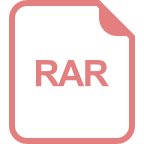
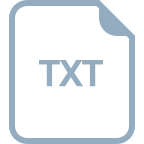
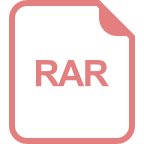
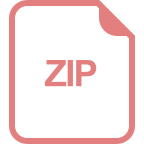
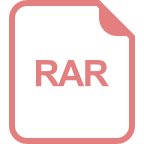
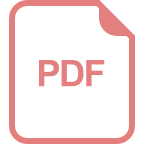


