c语言中大小写变换的代码
时间: 2023-04-08 12:04:37 浏览: 82
以下是将字符串中的大写字母转换为小写字母的C语言代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[] = "Hello World";
int i;
for (i = 0; str[i]; i++) {
str[i] = tolower(str[i]);
}
printf("%s\n", str);
return 0;
}
```
以上代码将输出:"hello world"。
相关问题
写一个c语言char型语句大小写变换的代码
以下是将输入字符串中的大写字母转换为小写字母,小写字母转换为大写字母的C语言代码:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void convert(char *str) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (isupper(str[i])) {
str[i] = tolower(str[i]);
} else if (islower(str[i])) {
str[i] = toupper(str[i]);
}
}
}
int main() {
char str[100];
printf("请输入一个字符串: ");
scanf("%s", str);
convert(str);
printf("转换后的字符串为: %s\n", str);
return 0;
}
```
在这个程序中,我们使用了C标准库中的两个函数:`isupper()`和`islower()`用于判断字符是否为大写字母或小写字母,`toupper()`和`tolower()`用于将字符转换为大写字母或小写字母。通过一个循环遍历字符串中的每一个字符,判断它的大小写并进行相应的转换,最终输出转换后的字符串。
c语言如何实现字符串大小写变换
C语言中,可以使用库函数toupper()和tolower()来实现字符串大小写变换。
toupper()函数将小写字母转换为大写字母,toupper()函数原型如下:
int toupper(int c);
其中,c表示待转换的字符,函数返回值是转换后的字符。
tolower()函数则将大写字母转换为小写字母,tolower()函数原型如下:
int tolower(int c);
同样,c为待转换的字符,函数返回值是转换后的字符。
使用这两个函数,我们可以实现字符串的大小写转换,例如:
#include <stdio.h>
#include <ctype.h>
int main()
{
char str[] = "Hello, World!";
int i = 0;
while (str[i])
{
str[i] = tolower(str[i]);
i++;
}
printf("%s\n", str);
return 0;
}
运行结果为:
hello, world!
以上代码中,我们先声明了一个字符串str,并使用while循环遍历每个字符。在循环内部,使用tolower()函数将每一个字符转换为小写字母。最后,我们输出转换后的字符串。
阅读全文
相关推荐




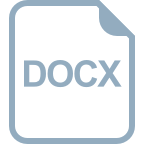
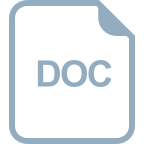
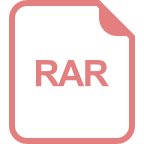
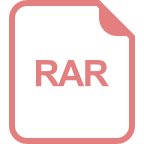





