ValueError: Unknown `data_format`: tf. Expected one of {'channels_first', 'channels_last'}
时间: 2023-12-29 18:05:25 浏览: 125
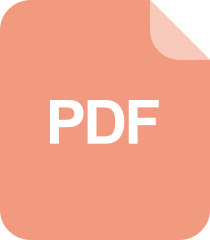
python学习笔记-面向对象高级编程-20200324
这个错误通常是由于你的代码中使用了TensorFlow的数据格式`'tf'`,但是Keras只支持`'channels_first'`和`'channels_last'`这两种数据格式。
要解决这个错误,需要将代码中使用的数据格式更改为`'channels_first'`或`'channels_last'`之一。通常情况下,你应该使用与你的Keras后端相对应的格式。
例如,如果你使用的是TensorFlow后端,你应该将数据格式设置为`'channels_last'`。你可以使用以下代码将数据格式设置为`'channels_last'`:
```
from keras import backend as K
K.set_image_data_format('channels_last')
```
如果你使用的是Theano后端,你应该将数据格式设置为`'channels_first'`。你可以使用以下代码将数据格式设置为`'channels_first'`:
```
from keras import backend as K
K.set_image_data_format('channels_first')
```
确保在你的代码中使用的所有模块中都使用相同的数据格式。
阅读全文
相关推荐
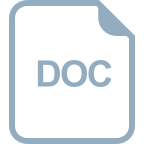
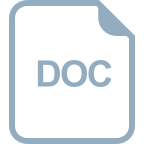
















