1、用程序实现波兰符号法。 实现功能:输入波兰符号法公式,求出公式类型 输入:波兰符号法的数学计算公式或命题公式 输出:输出公式类型。 输入公式的符号说明: 序号 联结词 程序替代符号 1 ¬ ! 2 ∧ & 3 ∨ | 4 → - 5 ↔ = 6 () ()
时间: 2024-02-23 20:01:43 浏览: 164
好的,以下是 Python 代码,用于实现波兰符号法的计算和公式类型的判断:
```python
# 定义运算符对应的程序替代符号
operators = {
'¬': '!',
'∧': '&',
'∨': '|',
'→': '-',
'↔': '=',
}
# 定义函数,用于将中缀表达式转换为后缀表达式
def infix_to_postfix(expression):
# 定义运算符的优先级
precedence = {
'!': 3,
'&': 2,
'|': 1,
'-': 0,
'=': 0,
}
stack = []
postfix = ''
for char in expression:
if char.isalpha():
postfix += char
elif char == '(':
stack.append(char)
elif char == ')':
while stack[-1] != '(':
postfix += stack.pop()
stack.pop()
else:
while stack and stack[-1] != '(' and precedence[char] <= precedence.get(stack[-1], -1):
postfix += stack.pop()
stack.append(char)
while stack:
postfix += stack.pop()
return postfix
# 定义函数,用于判断公式类型
def get_formula_type(expression):
postfix = infix_to_postfix(expression)
operators_count = 0
operands_count = 0
for char in postfix:
if char in operators.values():
operators_count += 1
elif char.isalpha():
operands_count += 1
if operators_count == 1 and operands_count == 2:
return '命题公式'
elif operators_count > 0 and operands_count > 0:
return '数学计算公式'
else:
return '不是公式'
# 测试
expression = input('请输入波兰符号法公式:')
expression = expression.replace(' ', '') # 去除空格
expression = expression.translate(str.maketrans(operators)) # 将符号替换为程序替代符号
formula_type = get_formula_type(expression)
print('公式类型为:', formula_type)
```
在测试时,可以输入如下格式的波兰符号法公式:
命题公式:!p
数学计算公式:+ 1 2
其中,命题公式只包含一个运算符和两个变量,而数学计算公式可以包含多个运算符和操作数。
阅读全文
相关推荐
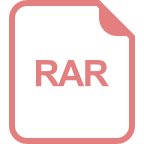



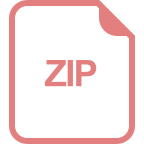
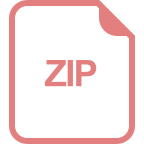
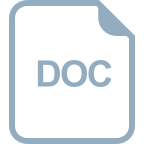
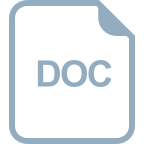
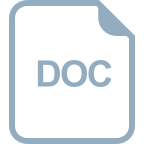
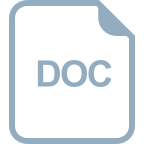
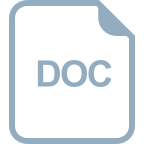
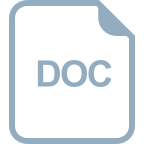
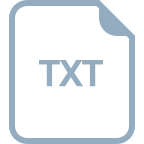
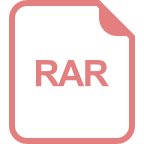
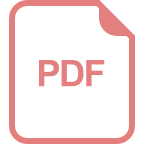
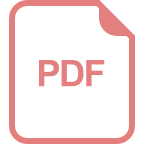