theadd() { if (this.addname == '' || this.addhosvalue == '') return this.$message.error('请填写完整信息') let data = { content: this.addname, paper_id: this.addhosvalue, qutype: this.radio, option1: this.option1, option2: this.option2, option3: this.option3, option4: this.option4, option5: this.option5, } this.$http.post(`admin/qu/questionAdd`, data).then(res => { if (res.data.code == 200) { this.$message.success('添加成功') this.getInfo() this.addutw = false } else { this.$message.error(res.data.msg) } }) },
时间: 2023-06-18 22:08:47 浏览: 149
这段代码是一个 Vue.js 中的方法,用于向服务器发送 POST 请求来添加一个问题。首先,它会检查 `addname` 和 `addhosvalue` 是否为空,如果有任何一个为空,则会弹出一个错误提示信息。然后,它会构造一个包含问题相关信息的数据对象 `data`,包括问题内容 `content`,所属试卷 ID `paper_id`,问题类型 `qutype`,以及选项 `option1` 到 `option5`。最后,它会使用 Vue.js 中的 `$http` 对象来发送 POST 请求,并根据服务器的响应结果弹出相应的提示信息。如果添加成功,则会刷新页面并关闭添加问题的窗口。
相关问题
这段代码改成可调用函数 : /*! * ASP.NET SignalR JavaScript Library 2.4.3 * http://signalr.net/ * * Copyright (c) .NET Foundation. All rights reserved. * Licensed under the Apache License, Version 2.0. See License.txt in the project root for license information. * */ /// <reference path="....\SignalR.Client.JS\Scripts\jquery-1.6.4.js" /> /// <reference path="jquery.signalR.js" /> (function ($, window, undefined) { /// <param name="$" type="jQuery" /> "use strict"; if (typeof ($.signalR) !== "function") { throw new Error("SignalR: SignalR is not loaded. Please ensure jquery.signalR-x.js is referenced before ~/signalr/js."); } var signalR = $.signalR; function makeProxyCallback(hub, callback) { return function () { // Call the client hub method callback.apply(hub, $.makeArray(arguments)); }; } function registerHubProxies(instance, shouldSubscribe) { var key, hub, memberKey, memberValue, subscriptionMethod; for (key in instance) { if (instance.hasOwnProperty(key)) { hub = instance[key]; if (!(hub.hubName)) { // Not a client hub continue; } if (shouldSubscribe) { // We want to subscribe to the hub events subscriptionMethod = hub.on; } else { // We want to unsubscribe from the hub events subscriptionMethod = hub.off; } // Loop through all members on the hub and find client hub functions to subscribe/unsubscribe for (memberKey in hub.client) { if (hub.client.hasOwnProperty(memberKey)) { memberValue = hub.client[memberKey]; if (!$.isFunction(memberValue)) { // Not a client hub function continue; } // Use the actual user-provided callback as the "identity" value for the registration. subscriptionMethod.call(hub, memberKey, makeProxyCallback(hub, memberValue), memberValue); } } } } } $.hubConnection.prototype.createHubProxies = function () { var proxies = {}; this.starting(function () { // Register the hub proxies as subscribed // (instance, shouldSubscribe) registerHubProxies(proxies, true); this._registerSubscribedHubs(); }).disconnected(function () { // Unsubscribe all hub proxies when we "disconnect". This is to ensure that we do not re-add functional call backs. // (instance, shouldSubscribe) registerHubProxies(proxies, false); }); proxies['realDataHub'] = this.createHubProxy('realDataHub'); proxies['realDataHub'].client = { }; proxies['realDataHub'].server = { send: function (name, message) { return proxies['realDataHub'].invoke.apply(proxies['realDataHub'], $.merge(["Send"], $.makeArray(arguments))); } }; return proxies; }; signalR.hub = $.hubConnection("/signalr", { useDefaultPath: false }); $.extend(signalR, signalR.hub.createHubProxies()); }(window.jQuery, window));
您可以将上述代码封装成一个可调用函数,例如:
```javascript
function connectToHub() {
if (typeof ($.signalR) !== "function") {
throw new Error("SignalR: SignalR is not loaded. Please ensure jquery.signalR-x.js is referenced before ~/signalr/js.");
}
var signalR = $.signalR;
var hubConnection = signalR.hubConnection("/signalr", { useDefaultPath: false });
var proxies = {};
function makeProxyCallback(hub, callback) {
return function () {
callback.apply(hub, $.makeArray(arguments));
};
}
function registerHubProxies(instance, shouldSubscribe) {
var key, hub, memberKey, memberValue, subscriptionMethod;
for (key in instance) {
if (instance.hasOwnProperty(key)) {
hub = instance[key];
if (!(hub.hubName)) {
continue;
}
if (shouldSubscribe) {
subscriptionMethod = hub.on;
} else {
subscriptionMethod = hub.off;
}
for (memberKey in hub.client) {
if (hub.client.hasOwnProperty(memberKey)) {
memberValue = hub.client[memberKey];
if (!$.isFunction(memberValue)) {
continue;
}
subscriptionMethod.call(hub, memberKey, makeProxyCallback(hub, memberValue), memberValue);
}
}
}
}
}
hubConnection.starting(function () {
registerHubProxies(proxies, true);
this._registerSubscribedHubs();
}).disconnected(function () {
registerHubProxies(proxies, false);
});
proxies['realDataHub'] = hubConnection.createHubProxy('realDataHub');
proxies['realDataHub'].client = {};
proxies['realDataHub'].server = {
send: function (name, message) {
return proxies['realDataHub'].invoke.apply(proxies['realDataHub'], $.merge(["Send"], $.makeArray(arguments)));
}
};
return proxies;
}
```
然后您可以在需要连接 SignalR 的地方调用该函数,例如:
```javascript
var hubProxies = connectToHub();
hubProxies.realDataHub.server.send('John', 'Hello!');
```
Also create a ControllerMoreBook class that extends Controller.The moreBook method takes the name of a user and a number of books (as a string) as arguments. The moreBook method of the controller then transforms the number of books from a string to an integer (using the Integer.parseInt static method) and calls the moreBook method of the library to increase the number of books borrowed or lent by the user (depending on what kind of user it is) of a specific user, by the given argument. • If no exception occurs then the moreBook method of the controller returns the empty string. • If the moreBook method of the library throws an UnknownUserException then the moreBook method of the controller must catch this exception and return as result the error message from the exception object. • If the moreBook method of the library throws a NotALenderException then the moreBook method of the controller must catch this exception and return as result the error message from the exception object. • If the parseInt method of the Integer class throws a NumberFormatException (because the user typed something which is not an integer) then the moreBook method of the controller must catch this exception and return as result the error message from the exception object. Note: to keep things simple, it is allowed for a user of your system to increase the number of books of a user by a negative number, so there is no need to check for that. Modify the run method of the GUI class to add a ViewMoreBook view that uses a ControllerMoreBook controller and the same model as before (not a new model!) Do not delete the previous views. Run your GUI and check that you can correctly use the new view to increase the number of books for different users of your library (obviously your library must have some users in it to test this: see the last paragraph of Question 7). • Check that, when you increase a user’s book, the simple view is automatically correctly updated to show the new total number of borrowed books for all users of the library. • Also use the “get book” view to check that the user’s book value correctly changed. • Also check that increasing the book number of an unknown user correctly shows an error message.Also check that increasing the book of a user by a large negative number correctly shows an error message. Also check that trying to increase the book of a user by a number which is not an integer correctly shows an error message (do not worry about the content of the error message). 完成符合以上要求的java代码
Library.java:
```java
import java.util.HashMap;
import java.util.Map;
public class Library {
private Map<String, User> users;
public Library() {
users = new HashMap<>();
}
public void addUser(User user) {
users.put(user.getName(), user);
}
public void moreBook(String name, int num) throws UnknownUserException, NotALenderException {
User user = users.get(name);
if (user == null) {
throw new UnknownUserException(name);
}
if (!(user instanceof Lender)) {
throw new NotALenderException(name);
}
Lender lender = (Lender) user;
lender.moreBook(num);
}
public int getNumBorrowed(String name) throws UnknownUserException {
User user = users.get(name);
if (user == null) {
throw new UnknownUserException(name);
}
return user.getNumBorrowed();
}
}
```
User.java:
```java
public abstract class User {
private String name;
private int numBorrowed;
public User(String name) {
this.name = name;
numBorrowed = 0;
}
public String getName() {
return name;
}
public int getNumBorrowed() {
return numBorrowed;
}
public void setNumBorrowed(int numBorrowed) {
this.numBorrowed = numBorrowed;
}
}
```
Lender.java:
```java
public class Lender extends User {
public Lender(String name) {
super(name);
}
public void moreBook(int num) {
setNumBorrowed(getNumBorrowed() + num);
}
}
```
UnknownUserException.java:
```java
public class UnknownUserException extends Exception {
private String name;
public UnknownUserException(String name) {
this.name = name;
}
public String getMessage() {
return "Unknown user: " + name;
}
}
```
NotALenderException.java:
```java
public class NotALenderException extends Exception {
private String name;
public NotALenderException(String name) {
this.name = name;
}
public String getMessage() {
return name + " is not a lender";
}
}
```
ControllerMoreBook.java:
```java
public class ControllerMoreBook extends Controller {
private Library library;
public ControllerMoreBook(Library library) {
this.library = library;
}
public String moreBook(String name, String numStr) {
try {
int num = Integer.parseInt(numStr);
library.moreBook(name, num);
return "";
} catch (UnknownUserException e) {
return e.getMessage();
} catch (NotALenderException e) {
return e.getMessage();
} catch (NumberFormatException e) {
return "Invalid number format";
}
}
}
```
ViewMoreBook.java:
```java
import javax.swing.*;
import java.awt.*;
public class ViewMoreBook extends JFrame implements View {
private JTextField nameField;
private JTextField numField;
private JButton okButton;
private JTextArea resultArea;
private ControllerMoreBook controller;
public ViewMoreBook(ControllerMoreBook controller) {
this.controller = controller;
setTitle("More Books");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(3, 2));
panel.add(new JLabel("Name:"));
nameField = new JTextField();
panel.add(nameField);
panel.add(new JLabel("Number of Books:"));
numField = new JTextField();
panel.add(numField);
okButton = new JButton("OK");
panel.add(okButton);
resultArea = new JTextArea();
resultArea.setEditable(false);
panel.add(resultArea);
add(panel);
okButton.addActionListener(e -> {
String name = nameField.getText();
String numStr = numField.getText();
String result = controller.moreBook(name, numStr);
resultArea.setText(result);
});
}
}
```
修改 GUI 类的 run 方法:
```java
public void run() {
Library library = new Library();
library.addUser(new Lender("Alice"));
library.addUser(new Lender("Bob"));
library.addUser(new Borrower("Charlie"));
ControllerSimple simpleController = new ControllerSimple(library);
ViewSimple simpleView = new ViewSimple(simpleController);
simpleView.setVisible(true);
ControllerGetBook getBookController = new ControllerGetBook(library);
ViewGetBook getBookView = new ViewGetBook(getBookController);
getBookView.setVisible(true);
ControllerMoreBook moreBookController = new ControllerMoreBook(library);
ViewMoreBook moreBookView = new ViewMoreBook(moreBookController);
moreBookView.setVisible(true);
}
```
阅读全文
相关推荐
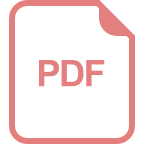
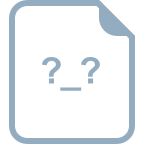
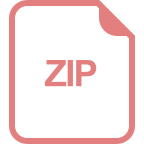
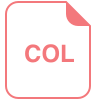
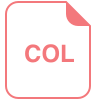











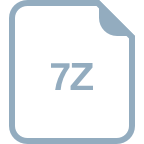