def __init__(self,input_dim,hidden_dim = 36): super(MLP, self).__init__()
时间: 2023-08-07 14:49:38 浏览: 146
该段代码是定义了一个名为MLP的类,它继承自PyTorch的nn.Module类,用于构建多层感知机模型。其中,__init__方法是类的构造函数,用于初始化MLP的参数。该方法中的input_dim参数表示输入数据的维度,hidden_dim参数表示隐藏层的维度,默认值为36。`super(MLP, self).__init__()`是Python中的super函数,用于调用父类的__init__()方法,这里是调用nn.Module类的构造函数进行初始化。这样,我们就可以在MLP类中使用nn.Module类中定义的方法和属性。
相关问题
import torch from torch import nn class MLP(nn.Module): def __init__(self, input_dim, num_class, hidden_dim) -> None: super().__init__() self.hidden_dim = hidden_dim self.mlp = nn.Sequential(*[ nn.Linear(input_dim, self.hidden_dim), nn.ReLU(), nn.Linear(self.hidden_dim, self.hidden_dim), nn.ReLU(), nn.Linear(self.hidden_dim, self.hidden_dim), nn.ReLU(), nn.Linear(self.hidden_dim, num_class) ]) def forward(self, x): return self.mlp(x)
这是一个使用 PyTorch 实现的多层感知器 (MLP) 的模型定义代码。该 MLP 模型包括一个输入层、若干个隐藏层和一个输出层。其输入特征维度为 input_dim,输出类别数为 num_class,隐藏层维度为 hidden_dim。其中,nn.Linear 表示全连接层,nn.ReLU 表示激活函数 ReLU。forward() 方法定义了模型的前向传播过程,即输入特征经过多个全连接层和激活函数后得到输出。在模型训练时,可以通过调用 forward() 方法来计算模型的输出,并根据输出与真实标签之间的差别来更新模型参数。
class MLP(nn.Module): def __init__( self, input_size: int, output_size: int, n_hidden: int, classes: int, dropout: float, normalize_before: bool = True ): super(MLP, self).__init__() self.input_size = input_size self.dropout = dropout self.n_hidden = n_hidden self.classes = classes self.output_size = output_size self.normalize_before = normalize_before self.model = nn.Sequential( nn.Linear(self.input_size, n_hidden), nn.Dropout(self.dropout), nn.ReLU(), nn.Linear(n_hidden, self.output_size), nn.Dropout(self.dropout), nn.ReLU(), ) self.after_norm = torch.nn.LayerNorm(self.input_size, eps=1e-5) self.fc = nn.Sequential( nn.Dropout(self.dropout), nn.Linear(self.input_size, self.classes) ) self.output_layer = nn.Linear(self.output_size, self.classes) def forward(self, x): self.device = torch.device('cuda') # x = self.model(x) if self.normalize_before: x = self.after_norm(x) batch_size, length, dimensions = x.size(0), x.size(1), x.size(2) output = self.model(x) return output.mean(dim=1) class LabelSmoothingLoss(nn.Module): def __init__(self, size: int, smoothing: float, ): super(LabelSmoothingLoss, self).__init__() self.size = size self.criterion = nn.KLDivLoss(reduction="none") self.confidence = 1.0 - smoothing self.smoothing = smoothing def forward(self, x: torch.Tensor, target: torch.Tensor) -> torch.Tensor: batch_size = x.size(0) if self.smoothing == None: return nn.CrossEntropyLoss()(x, target.view(-1)) true_dist = torch.zeros_like(x) true_dist.fill_(self.smoothing / (self.size - 1)) true_dist.scatter_(1, target.view(-1).unsqueeze(1), self.confidence) kl = self.criterion(torch.log_softmax(x, dim=1), true_dist) return kl.sum() / batch_size
这段代码中定义了一个 MLP 模型以及一个 LabelSmoothingLoss 损失函数。MLP 模型包含了多个线性层和 ReLU 激活函数,以及一个 LayerNorm 层和一个 dropout 层。LabelSmoothingLoss 损失函数主要用于解决分类问题中的过拟合问题,它通过对真实标签进行平滑处理来减少模型对噪声的敏感度。这段代码的 forward 方法实现了 MLP 模型的前向传播,以及 LabelSmoothingLoss 的计算。其中,true_dist 是经过平滑处理后的真实标签分布,kl 是计算 KL 散度的结果,最终返回的是 kl 的平均值。
阅读全文
相关推荐
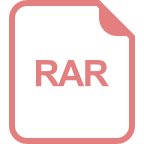
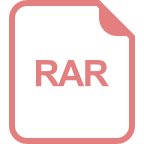
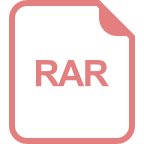












