设计一个图形绘制系统,包含抽象类Shape和具体的子类Circle(圆形)、Rectangle(矩形)。每个图形类有计算面积的方法。
时间: 2024-09-21 09:10:08 浏览: 56
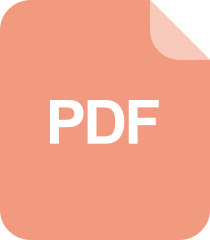
c++ 形状类Shape(派生出圆类Circle和矩形类Rectangle)
设计一个图形绘制系统,首先我们需要创建一个抽象类`Shape`,它作为所有图形的基础,定义共有的属性和方法,例如颜色、位置等。`Shape`类可能包含以下关键部分:
```java
abstract class Shape {
protected String color;
protected double positionX;
protected double positionY;
// 共享的行为
abstract double getArea();
// 其他可能的方法
public void setColor(String color) {
this.color = color;
}
public void setPosition(double x, double y) {
positionX = x;
positionY = y;
}
// 用于打印图形信息的通用方法
public abstract String toString();
}
```
然后,我们为`Circle`和`Rectangle`这两个具体形状创建子类,并覆盖`getArea()`方法:
```java
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
double getArea() {
return Math.PI * radius * radius;
}
@Override
public String toString() {
return "Circle [color=" + color + ", position=(" + positionX + ", " + positionY + "), radius=" + radius + "]";
}
}
class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
double getArea() {
return width * height;
}
@Override
public String toString() {
return "Rectangle [color=" + color + ", position=(" + positionX + ", " + positionY + "), width=" + width + ", height=" + height + "]";
}
}
```
现在,你可以创建这些形状的实例并计算它们的面积,同时利用多态的优势,不需要关心具体是哪种形状:
```java
public static void main(String[] args) {
Shape circle = new Circle(5);
Shape rectangle = new Rectangle(4, 6);
System.out.println("Circle area: " + circle.getArea());
System.out.println("Rectangle area: " + rectangle.getArea());
// 输出:
// Circle area: 78.53981633974483
// Rectangle area: 24
}
```
阅读全文
相关推荐
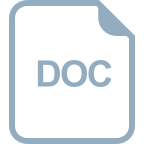
















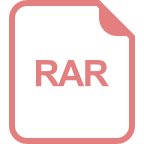
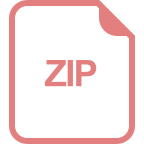