生成一个合并excel表的python 代码
时间: 2023-05-24 08:01:14 浏览: 88
非常感谢你的提问!以下是一个例子,可以帮助你合并多个Excel表格,可以根据需要进行修改:
```
import pandas as pd
import os
# 设置工作目录和文件扩展名
folder_path = 'your/folder/path/here'
extension = '.xlsx'
# 获取路径中所有excel文件的列表
os.chdir(folder_path)
all_filenames = [i for i in os.listdir() if i.endswith(extension)]
# 使用pandas库的concat函数合并Excel文件
combined_excel = pd.concat([pd.read_excel(f) for f in all_filenames ])
# 将合并后的数据保存为一个新的Excel文件
combined_excel.to_excel("combined_excel.xlsx", index=False, encoding='utf-8-sig')
```
希望这可以帮助到你!
相关问题
生成python合并多个excel文件代码
以下是一个合并多个excel文件的Python代码示例:
```python
import pandas as pd
import os
# 设置输入和输出文件夹路径
input_folder = "input/"
output_folder = "output/"
# 创建一个空的数据帧,用于存储所有excel文件的数据
merged_data = pd.DataFrame()
# 遍历输入文件夹中的所有excel文件
for file_name in os.listdir(input_folder):
if file_name.endswith(".xlsx"):
# 读取excel文件的数据
file_path = os.path.join(input_folder, file_name)
data = pd.read_excel(file_path)
# 将数据添加到merged_data中
merged_data = merged_data.append(data, ignore_index=True)
# 将合并的数据写入输出文件夹中的一个新excel文件
output_file_path = os.path.join(output_folder, "merged_data.xlsx")
merged_data.to_excel(output_file_path, index=False)
```
在此示例中,我们使用pandas库来读取和处理excel文件。我们首先设置输入和输出文件夹的路径,然后创建一个空的数据帧来存储所有excel文件的数据。我们使用os库中的listdir函数遍历输入文件夹中的所有excel文件,然后使用pandas的read_excel函数读取每个文件的数据,并将其添加到merged_data中。最后,我们使用to_excel函数将合并的数据写入一个新的excel文件中。
生成一个合并excel的程序
好的,以下是一个 Python 实现的合并 Excel 文件的程序:
```python
import pandas as pd
# 读取所有要合并的 Excel 文件
excel_files = ['file1.xlsx', 'file2.xlsx', 'file3.xlsx']
df_list = [pd.read_excel(file) for file in excel_files]
# 合并所有数据
merged_df = pd.concat(df_list)
# 将合并后的数据保存到一个新的 Excel 文件中
merged_df.to_excel('merged_file.xlsx', index=False)
```
上面的代码中,我们首先定义了要合并的 Excel 文件的文件名,然后使用 Pandas 库中的 `read_excel` 函数读取所有文件。接着,使用 Pandas 库中的 `concat` 函数将所有数据合并到一个 DataFrame 中。最后,将合并后的数据保存到一个新的 Excel 文件中。
需要注意的是,上面的代码中假设所有 Excel 文件的结构相同,即列名和列数相同。如果不同,需要根据具体情况进行修改。
阅读全文
相关推荐
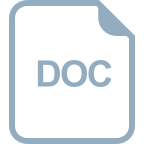
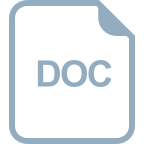
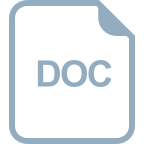





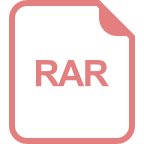
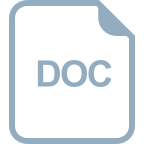





