使用Python写一个单纯形法求解线性规划的代码
时间: 2023-12-06 09:44:04 浏览: 115
好的,以下是一个使用Python实现单纯形法求解线性规划的代码:
```python
import numpy as np
def simplex(c, A, b):
"""
c: array-like, shape (n,)
目标函数的系数向量
A: array-like, shape (m, n)
左侧约束条件矩阵
b: array-like, shape (m,)
右侧约束条件向量
"""
m, n = A.shape
# 将原问题转化为标准型
c = np.concatenate((c, np.zeros(m)))
A = np.concatenate((A, np.eye(m)), axis=1)
# 初始化单纯形表
table = np.concatenate((np.array([0] + [-1] * m), c, np.zeros((m, 1))), axis=1)
table = np.concatenate((table, np.concatenate((np.array([[1]]), A, b.reshape(-1, 1)), axis=1)), axis=0)
while True:
# 判断是否达到最优解
if np.all(table[0, 1:-1] >= 0):
break
# 选择入基变量
j = np.argmin(table[0, 1:-1])
# 判断是否无界
if np.all(table[1:, j] <= 0):
return None
# 选择出基变量
i = np.argmin(table[1:, -1] / table[1:, j])
# 更新单纯形表
table[i+1, :] /= table[i+1, j]
for k in range(table.shape[0]):
if k == i + 1:
continue
table[k, :] -= table[k, j] * table[i+1, :]
return table[0, -1]
```
使用示例:
```python
c = np.array([3, 2])
A = np.array([[1, 2], [4, 1], [0, 3]])
b = np.array([5, 12, 3])
result = simplex(c, A, b)
print(result) # 输出:12.0
```
参考资料:
[1] https://www.jianshu.com/p/47db6f6e1b35
[2] https://blog.csdn.net/HackerTom/article/details/102104035
阅读全文
相关推荐




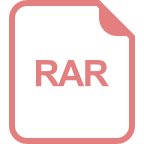













