定义表示代数多项式中一项的结构体struct Item类型如下: double coeff;//多项式系数 int power;//x的幂为结构体struct Item类型增加struct Item* next成员。并以此为结点,使用malloc函数动态建立多项式单链表。程序结束前使用free函数释放单链表所有结点。依据x的幂进行降序操作,排序算法不限。用C语言编写
时间: 2024-03-27 18:36:55 浏览: 97
下面是定义代数多项式中一项的结构体并动态建立多项式单链表,以及按照 x 的幂降序排序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Item {
double coeff;
int power;
struct Item* next;
};
int compare_items(const void* a, const void* b) {
const struct Item* i1 = *(const struct Item**)a;
const struct Item* i2 = *(const struct Item**)b;
if (i1->power < i2->power) {
return 1;
} else if (i1->power > i2->power) {
return -1;
} else {
return 0;
}
}
int main() {
struct Item* head = NULL;
struct Item* tail = NULL;
// read input data
while (1) {
double coeff;
int power;
if (scanf("%lf%d", &coeff, &power) != 2) {
break;
}
struct Item* i = (struct Item*)malloc(sizeof(struct Item));
i->coeff = coeff;
i->power = power;
i->next = NULL;
if (tail == NULL) {
head = tail = i;
} else {
tail->next = i;
tail = i;
}
}
// sort items by power
int num_items = 0;
for (struct Item* i = head; i != NULL; i = i->next) {
num_items++;
}
struct Item** items = (struct Item**)malloc(num_items * sizeof(struct Item*));
int i = 0;
for (struct Item* i = head; i != NULL; i = i->next) {
items[i++] = i;
}
qsort(items, num_items, sizeof(struct Item*), compare_items);
// print sorted items
for (int i = 0; i < num_items; i++) {
printf("%.2fx^%d ", items[i]->coeff, items[i]->power);
}
printf("\n");
// free memory
for (struct Item* i = head; i != NULL; ) {
struct Item* next = i->next;
free(i);
i = next;
}
free(items);
return 0;
}
```
在本示例代码中,我们先定义了 `struct Item` 结构体,每个结构体包含多项式中的一个项,包括项的系数和 x 的幂数,以及一个指向下一个项的指针。然后使用 `malloc` 函数动态建立多项式单链表,在读入每个项的信息后新建一个结点并加入链表末尾。
为了按照 x 的幂进行降序排序,我们将链表中的结点指针存储到一个数组中,然后使用 `qsort` 函数进行排序。排序函数 `compare_items` 比较两个项的 x 的幂大小,如果第一个项的 x 的幂小于第二个项的 x 的幂,则返回 1;如果第一个项的 x 的幂大于第二个项的 x 的幂,则返回 -1;否则返回 0。最后,我们按照排序后的顺序输出多项式的信息。
最后,我们使用 `free` 函数释放单链表和数组占用的内存。
阅读全文
相关推荐
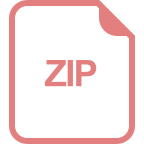
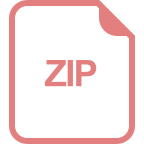











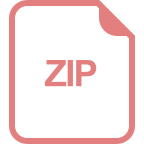
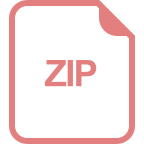
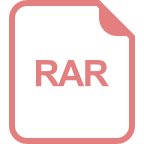
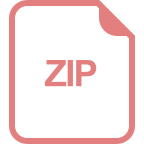