用c语言设计线性链表求一元多项式的导数要求以指数递降方式输入多项式非零项系数和指数(绝对值不超过1000的整数)数字间以空格分隔,以与输入相同的格式输出导数多项式非零项的系数和指数数字间以空格分隔但结尾不能有多余空格
时间: 2024-10-22 18:22:18 浏览: 35
在C语言中设计一个函数来计算一元多项式的导数,我们需要首先理解多项式的基本形式。一个一元多项式可以表示为:
\[ p(x) = a_n x^n + a_{n-1}x^{n-1} + ... + a_1x + a_0 \]
其中,\( a_i \) 是系数,\( n \) 是最高次幂的指数。
为了实现这个功能,我们可以创建一个结构体来存储每一项的系数和指数,并遍历链表来计算导数。导数公式为:
\[ p'(x) = na_n x^{n-1} + (n-1)a_{n-1}x^{n-2} + ... + a_1 \]
下面是一个简单的链表数据结构和计算导数的函数示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int coefficient;
int exponent;
struct Node *next;
} Node;
Node* createNode(int coeff, int exp) {
Node *newNode = malloc(sizeof(Node));
newNode->coefficient = coeff;
newNode->exponent = exp;
newNode->next = NULL;
return newNode;
}
void insertNode(Node **head, int coeff, int exp) {
if (*head == NULL) {
*head = createNode(coeff, exp);
} else {
Node *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = createNode(coeff, exp);
}
}
Node* derivative(Node *head) {
if (head == NULL || head->next == NULL) return head; // 如果只有一个常数项,则导数为0
Node *derivativeHead = createNode(head->exponent * head->coefficient, head->exponent - 1); // 新链表的第一项
Node *current = head->next;
while (current != NULL) {
Node *temp = createNode(current->exponent * current->coefficient, current->exponent - 1);
temp->next = derivativeHead->next;
derivativeHead->next = temp;
current = current->next;
}
return derivativeHead;
}
void printPolynomial(Node *head) {
while (head != NULL) {
printf("%d %d ", head->coefficient, head->exponent);
head = head->next;
}
printf("\n");
}
int main() {
Node *poly = NULL;
char input[1001];
gets(input); // 注意这里只是一个简化示例,实际程序需要处理输入错误
const char *end = strchr(input, ' ');
int coeff = atoi(end + 1), exp = 0; // 假设输入为 "coeff space exp"
insertNode(&poly, coeff, exp);
end += strlen(end + 1) + 1;
while (end < input + strlen(input)) {
coeff = atoi(end);
end = strchr(end + 1, ' ');
exp = atoi(end + 1);
insertNode(&poly, coeff, exp);
}
printf("Original polynomial: ");
printPolynomial(poly);
poly = derivative(poly);
printf("Derivative: ");
printPolynomial(poly);
return 0;
}
```
这个程序首先读取用户输入的一元多项式的系数和指数,然后计算并打印出导数。注意,上述代码仅作示例,实际应用中你需要增加错误处理和输入验证部分。
阅读全文
相关推荐
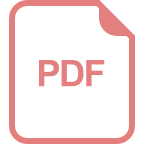
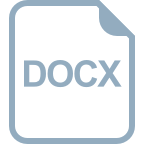
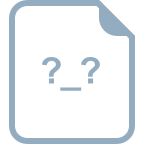











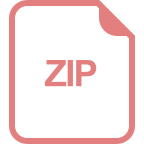
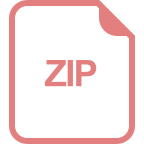