使用c语言设计程序求一元多项式的导数。 输入格式: 输入一共2行,第一行是多项式的项数,第二行给出每一项的系数和指数。系数和指数都是绝对值不超过1000的整数。系数不可能为0。 输出格式: 输出多项式求导后每一项的系数和指数,中间用空格隔开,但结尾不能有多余空格。指数递增有序
时间: 2024-09-27 12:08:34 浏览: 66
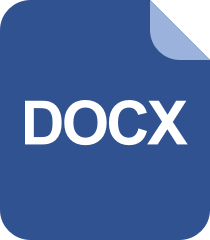
数据结构历次算法总结.docx
在C语言中,为了计算一元多项式的导数,你可以使用函数来处理每个项并应用导数规则。对于n次多项式 `a_n * x^n + a_{n-1} * x^(n-1) + ... + a_1 * x^1 + a_0`,其导数是 `n*a_n * x^(n-1) + (n-1)*a_{n-1} * x^(n-2) + ... + a_1`。下面是一个简单的示例函数,用于实现这个过程:
```c
#include <stdio.h>
// 函数声明,假设有一个结构体存储系数和指数
typedef struct {
int coefficient;
int exponent;
} Term;
void derivative(Term* terms, int n) {
for (int i = 1; i < n; i++) { // 开始从x^1到x^(n-1)
terms[i].coefficient *= i;
terms[i].exponent -= 1;
}
}
// 主函数,读取输入,计算导数并输出结果
int main() {
int num_terms;
scanf("%d", &num_terms);
Term* polynomial = malloc(num_terms * sizeof(Term));
for (int i = 0; i < num_terms; i++) {
scanf("%d %d", &polynomial[i].coefficient, &polynomial[i].exponent);
if (i > 0 && polynomial[i].exponent == polynomial[i - 1].exponent) {
printf("Error: Consecutive terms with the same exponent not supported.\n");
return 1; // 处理相同的指数项错误
}
}
derivative(polynomial, num_terms); // 计算导数
for (int i = 0; i < num_terms; i++) {
printf("%d %d ", polynomial[i].coefficient, polynomial[i].exponent); // 输出结果
}
free(polynomial); // 释放内存
printf("\n");
阅读全文
相关推荐
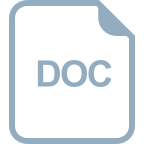
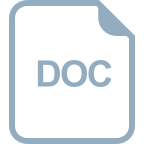







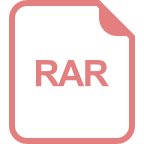
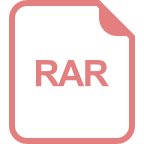






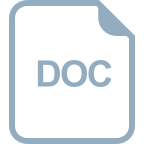