输入一个多项式如何求他的导数用c语言实现
时间: 2024-12-15 19:18:05 浏览: 5
在C语言中,为了求解多项式的导数,你需要对每个项应用幂规则,即对于形如f(x) = a_n * x^n + a_{n-1} * x^{n-1} + ... + a_1 * x^1 + a_0,其导数f'(x) = n * a_n * x^(n-1) + (n-1) * a_{n-1} * x^(n-2) + ... + 1 * a_1。这里的关键点是要能处理多项式系数和指数。
下面是一个简单的示例,展示如何递归地计算多项式的导数:
```c
#include <stdio.h>
// 定义多项式结构体
typedef struct {
double coefficient;
int exponent;
} Term;
// 添加一个新项到多项式
void addTerm(Term* poly, double coef, int exp) {
poly->coefficient *= exp;
poly++;
}
// 求导函数,假设poly指向多项式的最后一个元素
Term derivative(Term poly[]) {
if (poly[0].exponent == 0) return (Term){0, 0}; // 如果是常数项,导数为0
Term derivativeTerm;
derivativeTerm.coefficient = poly[0].coefficient * poly[0].exponent;
derivativeTerm.exponent -= 1; // 减去当前指数
poly++; // 移动到下一个项
return derivativeTerm;
}
int main() {
Term poly[] = {{2.0, 3}, {1.5, 2}, {0.5, 1}, {1.0, 0}}; // 例如多项式 f(x) = 2x^3 + 1.5x^2 + 0.5x + 1
Term derivativePoly[4]; // 存储导数的多项式
for (int i = 0; i < sizeof(poly) / sizeof(poly[0]); ++i) {
derivativePoly[i] = derivative(poly);
printf("f'(x) = %.2fx^%.0f + ", derivativePoly[i].coefficient, derivativePoly[i].exponent);
}
// 输出导数多项式,注意去掉最后的逗号
printf("\n");
return 0;
}
```
在这个例子中,我们首先创建了一个包含多项式各个项的数组,然后通过循环遍历每一项并调用`derivative`函数来更新导数多项式。记得在实际应用中根据需要添加错误检查和边界条件处理。
阅读全文
相关推荐
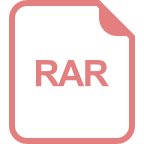
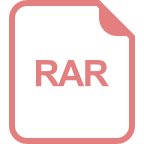
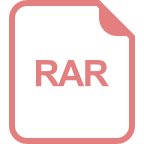















