def four_point_transform(image, pts): # obtain a consistent order of the points and unpack them # individually rect = order_points(pts) (tl, tr, br, bl) = rect # compute the width of the new image, which will be the # maximum distance between bottom-right and bottom-left # x-coordiates or the top-right and top-left x-coordinates widthA = np.sqrt(((br[0] - bl[0]) ** 2) + ((br[1] - bl[1]) ** 2)) widthB = np.sqrt(((tr[0] - tl[0]) ** 2) + ((tr[1] - tl[1]) ** 2)) maxWidth = max(int(widthA), int(widthB)) # compute the height of the new image, which will be the # maximum distance between the top-right and bottom-right # y-coordinates or the top-left and bottom-left y-coordinates heightA = np.sqrt(((tr[0] - br[0]) ** 2) + ((tr[1] - br[1]) ** 2)) heightB = np.sqrt(((tl[0] - bl[0]) ** 2) + ((tl[1] - bl[1]) ** 2)) maxHeight = max(int(heightA), int(heightB)) # now that we have the dimensions of the new image, construct # the set of destination points to obtain a "birds eye view", # (i.e. top-down view) of the image, again specifying points # in the top-left, top-right, bottom-right, and bottom-left # order dst = np.array([ [0, 0], [maxWidth - 1, 0], [maxWidth - 1, maxHeight - 1], [0, maxHeight - 1]], dtype = "float32") # compute the perspective transform matrix and then apply it M = cv2.getPerspectiveTransform(rect, dst) warped = cv2.warpPerspective(image, M, (maxWidth, maxHeight)) # return the warped image return warped
时间: 2023-06-18 19:02:32 浏览: 292
这是一个使用透视变换的函数,它可以将一个四边形区域从原图像中提取出来,并将其变换成一个矩形。主要步骤如下:
1. 对四个顶点进行排序,以确保它们按照左上、右上、右下、左下的顺序排列;
2. 计算新图像的宽度和高度,分别为原四边形区域的最大宽度和最大高度;
3. 构造目标顶点集合,将它们指定为矩形的左上、右上、右下、左下四个点;
4. 计算透视变换矩阵,并将其应用到原图像上,得到变换后的矩形图像;
5. 返回变换后的矩形图像。
这个函数可以用于许多应用,例如车牌识别、文档扫描等。
相关问题
解释def four_point_transform(image, pts): # 透视变换得到车牌小图 # rect = order_points(pts) rect = pts.astype('float32') (tl, tr, br, bl) = rect widthA = np.sqrt(((br[0] - bl[0]) ** 2) + ((br[1] - bl[1]) ** 2)) widthB = np.sqrt(((tr[0] - tl[0]) ** 2) + ((tr[1] - tl[1]) ** 2)) maxWidth = max(int(widthA), int(widthB)) heightA = np.sqrt(((tr[0] - br[0]) ** 2) + ((tr[1] - br[1]) ** 2)) heightB = np.sqrt(((tl[0] - bl[0]) ** 2) + ((tl[1] - bl[1]) ** 2)) maxHeight = max(int(heightA), int(heightB)) dst = np.array([ [0, 0], [maxWidth - 1, 0], [maxWidth - 1, maxHeight - 1], [0, maxHeight - 1]], dtype="float32") M = cv2.getPerspectiveTransform(rect, dst) warped = cv2.warpPerspective(image, M, (maxWidth, maxHeight)) return warped
这段代码是一个使用 OpenCV 库实现的透视变换函数,可以通过给定的四个点坐标将原图像中的一个矩形区域变换为一个矩形区域,可以用于车牌识别等应用场景。函数的输入参数为原图像和四个点的坐标,输出结果为变换后的小图像。该函数首先对输入的点进行顺时针排序,然后计算出目标矩形的宽度和高度,生成目标矩形的四个顶点坐标,接着通过 getPerspectiveTransform 函数计算透视变换矩阵,最后通过 warpPerspective 函数将原图像进行透视变换得到变换后的小图像。
pts_4d = torch.cat([points, points.new_ones(size=(num_points, 1))], dim=-1) pts_2d = pts_4d @ lidar2img_rt[view_idx].t() # pts_2d = pts_4d @ lidar2img_rt.t() pts_2d[:, 2] = torch.clamp(pts_2d[:, 2], min=1e-5) pts_2d[:, 0] /= pts_2d[:, 2] pts_2d[:, 1] /= pts_2d[:, 2] # img transformation: scale -> crop -> flip # the image is resized by img_scale_factor img_coors = pts_2d[:, 0:2] * img_scale_factor img_coors -= img_crop_offset
这段代码的作用是将点云数据从激光雷达坐标系转换到图像坐标系。具体来说,首先将点云坐标加上一个齐次坐标1,变成4维坐标。然后通过lidar2img_rt矩阵将点云坐标变换到图像坐标系下。接着将变换后的坐标系中的z轴坐标值进行限制,避免其小于1e-5。最后将x、y坐标值除以z坐标值,得到在图像中的坐标值。最后,将图像坐标系中的坐标进行缩放和平移,并进行图像的翻转操作。
阅读全文
相关推荐
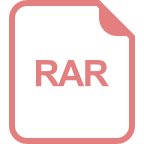













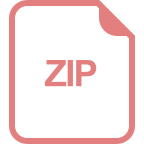