设计一个复数类,私有数据成员用float,要求可以用以下main函数调用。 int main() { Complex a, b, c, d, e, f; a.input(); b.input(); c = a + b; d = a - b; e = a * b; f = a / b; a.output(); b.output(); c.output(); d.output(); e.output(); f.output(); return 0; } 输入 输入一个复数的实部和虚部 输出 按样例输出结果 样例输入 Copy 1 2 3 4 样例输出 Copy 1+2i 3+4i 4+6i -2-2i -5+10i 0.44+0.08i
时间: 2024-03-15 10:45:56 浏览: 83
好的,以下是一个简单的复数类的实现,可以满足题目要求:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
float real, imag; // 实部和虚部
public:
Complex() {} // 默认构造函数
Complex(float r, float i) : real(r), imag(i) {} // 带参数构造函数
// 输入函数
void input() {
cin >> real >> imag;
}
// 输出函数
void output() {
cout << real << (imag >= 0 ? "+" : "") << imag << "i" << endl;
}
// 加法运算符重载
Complex operator+(const Complex& c2) const {
return Complex(real + c2.real, imag + c2.imag);
}
// 减法运算符重载
Complex operator-(const Complex& c2) const {
return Complex(real - c2.real, imag - c2.imag);
}
// 乘法运算符重载
Complex operator*(const Complex& c2) const {
return Complex(real * c2.real - imag * c2.imag, real * c2.imag + imag * c2.real);
}
// 除法运算符重载
Complex operator/(const Complex& c2) const {
float denominator = c2.real * c2.real + c2.imag * c2.imag;
return Complex((real * c2.real + imag * c2.imag) / denominator, (imag * c2.real - real * c2.imag) / denominator);
}
};
int main() {
Complex a, b, c, d, e, f;
a.input();
b.input();
c = a + b;
d = a - b;
e = a * b;
f = a / b;
a.output();
b.output();
c.output();
d.output();
e.output();
f.output();
return 0;
}
```
上述代码中,我们定义了一个名为 `Complex` 的类,它包括了私有数据成员 `real` 和 `imag`,表示复数的实部和虚部。我们在类中重载了加、减、乘、除四种运算符,使得可以通过 `+`、`-`、`*`、`/` 符号来进行复数的运算。在 `output` 函数中,我们根据实部和虚部的正负情况来输出复数的字符串表示。最后,在 `main` 函数中,我们调用了 `Complex` 类的各种成员函数来实现复数运算,并按照题目要求输出结果。
阅读全文
相关推荐
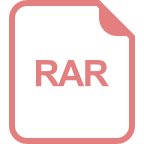
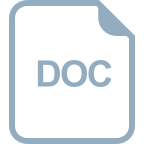

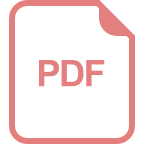
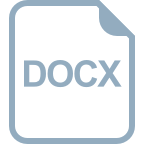
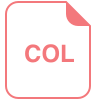
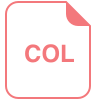


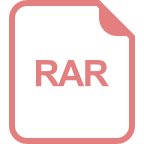
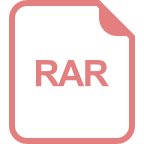
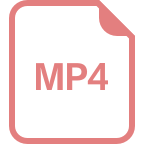
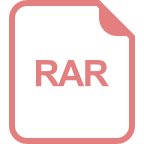
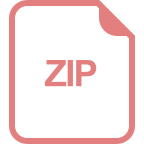