import jieba# 读取文件内容with open('春.txt', 'r', encoding='utf-8') as f: content = f.read()# 精确模式分词words = jieba.cut(content, cut_all=False)# 输出分词结果for word in words: print(word)简化一下这个代码
时间: 2023-12-09 14:04:31 浏览: 154
可以简化为以下代码:
```python
import jieba
with open('春.txt', 'r', encoding='utf-8') as f:
content = f.read()
words = jieba.lcut(content)
print(words)
```
其中,`jieba.lcut()` 函数是 `jieba.cut()` 函数的一个简化形式,使用时无需指定 `cut_all` 参数。同时,可以将分词结果直接输出为一个列表,方便查看。
相关问题
import jieba# 读取txt文件中的内容with open('input.txt', 'r', encoding='utf-8') as f: text = f.read()# 使用jieba进行分词seg_list = jieba.cut(text)# 将分词结果写入output.txt文件中with open('output.txt', 'w', encoding='utf-8') as f: f.write(' '.join(seg_list))
这段代码是使用jieba库对一个名为"input.txt"的文件进行分词,并将分词结果写入到"output.txt"文件中。具体流程为:首先使用Python的open函数读取"input.txt"文件中的内容,然后使用jieba库的cut函数对文本进行分词。分词结果是一个生成器,需要使用join函数将其转换为字符串,并在每个分词之间添加一个空格。最后,使用open函数创建一个名为"output.txt"的文件,并将分词结果写入其中。
#请上传程序文件。 #读取”三国人名汇总.txt”中的人物名字,读取“三国演义.txt”的全部内容 #先统计所有人物的名字在书本中出现的次数 #并对出现次数超过100次的人物绘制一个柱状图,如下图所示。 import jieba import matplotlib.pyplot as plt from collections import Counter# 读取人名列表 with open(r"C:\Users\asus\Desktop\三国演义数据文件\三国人名汇总.txt", 'r', encoding='utf-8') as f: names = [line.strip() for line in f.readlines()]# 读取三国演义内容 with open(r"C:\Users\asus\Desktop\三国演义数据文件\三国演义.txt", 'r', encoding='utf-8') as f: content = f.read()# 使用jieba分词对内容进行处理 words = list(jieba.cut(content))# 统计人名出现的次数 name_counts = Counter() for word in words: if word in names: name_counts[word] += 1# 绘制柱状图 top_names = [] name_counts = name_counts.most_common() for name, count in name_counts: if count > 100: top_names.append(name) plt.bar(name, count) plt.xticks(rotation=90) plt.title('Top Characters in Three Kingdoms') plt.xlabel('Name') plt.ylabel('Count') plt.show() 帮忙debug 帮忙不要用counter,用count函数
好的,这里给您一个不使用 Counter 的版本,使用 count 函数统计人名出现的次数:
```python
import jieba
import matplotlib.pyplot as plt
# 读取人名列表
with open(r"C:\Users\asus\Desktop\三国演义数据文件\三国人名汇总.txt", 'r', encoding='utf-8') as f:
names = [line.strip() for line in f.readlines()]
# 读取三国演义内容
with open(r"C:\Users\asus\Desktop\三国演义数据文件\三国演义.txt", 'r', encoding='utf-8') as f:
content = f.read()
# 使用jieba分词对内容进行处理
words = list(jieba.cut(content))
# 统计人名出现的次数
name_counts = {}
for name in names:
count = words.count(name)
if count > 0:
name_counts[name] = count
# 绘制柱状图
top_names = []
for name, count in name_counts.items():
if count > 100:
top_names.append(name)
plt.bar(name, count)
plt.xticks(rotation=90)
plt.title('Top Characters in Three Kingdoms')
plt.xlabel('Name')
plt.ylabel('Count')
plt.show()
```
这个版本使用了一个字典 `name_counts` 来存储人名及其出现的次数,然后使用 `items()` 遍历字典中的键值对进行统计。如果出现次数超过 100 次,则添加到 `top_names` 列表中。最后绘制柱状图。
阅读全文
相关推荐
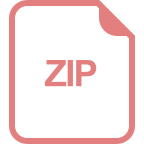















