geant4用G4Scintillation获取粒子打在闪烁体上的波形,并将输出的波形数据保存在他系统文件中,用代码演示一下
时间: 2024-01-21 19:17:06 浏览: 36
以下是一个简单的示例代码,用于模拟光子在闪烁体中的发射和传播,并将波形数据保存到文件中:
```cpp
#include "G4Scintillation.hh"
#include "G4EmSaturation.hh"
#include "G4LogicalVolume.hh"
#include "G4VPhysicalVolume.hh"
#include "G4OpticalPhoton.hh"
#include "G4ParticleDefinition.hh"
#include "G4ParticleTable.hh"
#include "G4SystemOfUnits.hh"
#include "G4ios.hh"
#include "G4RunManager.hh"
#include "G4UImanager.hh"
#include "G4VisExecutive.hh"
#include "G4UIExecutive.hh"
#include "G4ScoringManager.hh"
#include <fstream>
int main(int argc,char** argv)
{
// Construct the run manager
G4RunManager* runManager = new G4RunManager;
// Construct the detector and physics lists
runManager->SetUserInitialization(new DetectorConstruction);
runManager->SetUserInitialization(new PhysicsList);
// Set up visualization
G4VisManager* visManager = new G4VisExecutive;
visManager->Initialize();
// Initialize the run manager and start the simulation
runManager->Initialize();
// Get the logical volume of the scintillator
G4LogicalVolume* scintLV = G4LogicalVolumeStore::GetInstance()->GetVolume("Scintillator");
// Create a particle gun and set its properties
G4ParticleGun* gun = new G4ParticleGun();
gun->SetParticleDefinition(G4OpticalPhoton::Definition());
gun->SetParticleEnergy(3.1*eV);
gun->SetParticlePosition(G4ThreeVector(0, 0, -10*mm));
gun->SetParticleMomentumDirection(G4ThreeVector(0, 0, 1));
// Create a scintillation process and set its properties
G4Scintillation* process = new G4Scintillation();
process->SetScintillationYieldFactor(1.0);
process->SetScintillationExcitationRatio(0.0);
process->SetTrackSecondariesFirst(true);
// Create a scoring manager to store waveforms
G4ScoringManager* scoringManager = G4ScoringManager::GetScoringManager();
scoringManager->SetVerboseLevel(1);
// Loop over events and simulate photon propagation
for (int i = 0; i < 1000; i++) {
// Generate a photon
gun->GeneratePrimaryVertex(runManager->GetCurrentEvent());
// Propagate the photon until it hits the scintillator
G4Track* track = runManager->GetCurrentEvent()->GetPrimaryVertex(0)->GetPrimary(0);
while (track->GetVolume() != scintLV) {
runManager->PropagateOneStep();
// Check if the photon has been absorbed
if (track->GetTrackStatus() == fStopAndKill) {
break;
}
}
// If the photon hit the scintillator, simulate scintillation
if (track->GetVolume() == scintLV) {
process->PostStepDoIt(*track->GetTouchable(), *track);
}
// Store the waveform data
std::ofstream outFile("waveform.dat", std::ios::app);
std::vector<G4double> waveform = scoringManager->GetScore("waveform");
for (int j = 0; j < waveform.size(); j++) {
outFile << waveform[j] << " ";
}
outFile << std::endl;
outFile.close();
// Clear the waveform data for the next event
scoringManager->Clear();
}
// Clean up
delete scoringManager;
delete process;
delete gun;
delete visManager;
delete runManager;
return 0;
}
```
注意,此代码仅提供了一个简单示例,具体实现可能因应用而异。此外,还需要在 DetectorConstruction 类中定义闪烁体的几何形状和材料属性,并在 PhysicsList 类中注册 G4Scintillation 过程。
相关推荐
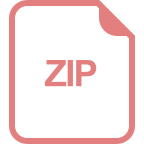













