import requests import re, os from lxml import etree headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/78.0.3904.108 Safari/537.36' } url = 'http://www.biquku.la/0/421/' def get_info(url): response = requests.get(url, headers=headers) response.encoding = 'utf-8' get_info_list = [] html = etree.HTML(response.text) dd_list = html.xpath('//*[@id="list"]/dl/dd') for dd in dd_list: title = dd.xpath('a/text()')[0] href = 'http://www.biquku.la/0/421/' + dd.xpath('a/@href')[0] chapter = {'title': title, 'href': href} get_info_list.append(chapter) return get_info_list def get_content(get_info): for chapter_info in get_info: response = requests.get(url=chapter_info['href'], headers=headers) response.encoding = 'utf-8' if os.path.exists('斗罗大陆'): pass else: os.makedirs('斗罗大陆') contents = re.findall('<div id="content">(.*?)</div>', response.text) with open('./斗罗大陆/' + chapter_info['title'] + '.txt', 'w', encoding='utf-8') as f: for content in contents: f.write(content.replace(' ', '').replace('<br/><br/>', '\n').strip()) print('下载成功') if __name__ == '__main__': get_content(get_info(url))帮我解释一下这个python代码每行的意思是什么
时间: 2024-04-28 19:22:51 浏览: 17
这段 Python 代码主要是爬取小说网站上的小说《斗罗大陆》的每个章节内容,并保存为文本文件。下面是对每行代码的解释:
1. `import requests import re, os from lxml import etree`:导入所需的 Python 模块。
2. `headers = {...}`:设置请求头,模拟浏览器请求。
3. `url = 'http://www.biquku.la/0/421/'`:目标小说的网址。
4. `def get_info(url):`:定义一个函数,获取小说的章节目录。
5. `response = requests.get(url, headers=headers)`:向目标网址发送请求。
6. `response.encoding = 'utf-8'`:设置响应的编码格式。
7. `get_info_list = []`:创建一个空列表,用于存储章节目录信息。
8. `html = etree.HTML(response.text)`:使用 lxml 库的 etree 模块对响应内容进行解析。
9. `dd_list = html.xpath('//*[@id="list"]/dl/dd')`:使用 xpath 解析出章节目录所在的 HTML 元素。
10. `for dd in dd_list: ...`:循环遍历每一个章节链接。
11. `title = dd.xpath('a/text()')[0]`:使用 xpath 解析出章节的标题。
12. `href = 'http://www.biquku.la/0/421/' + dd.xpath('a/@href')[0]`:使用 xpath 解析出章节的链接。
13. `chapter = {'title': title, 'href': href}`:将章节的标题和链接保存为一个字典。
14. `get_info_list.append(chapter)`:将字典添加到列表中。
15. `return get_info_list`:返回包含所有章节信息的列表。
16. `def get_content(get_info):`:定义一个函数,获取每个章节的内容。
17. `for chapter_info in get_info:`:循环遍历每一个章节的信息。
18. `response = requests.get(url=chapter_info['href'], headers=headers)`:向章节链接发送请求。
19. `response.encoding = 'utf-8'`:设置响应的编码格式。
20. `if os.path.exists('斗罗大陆'):`:判断当前目录下是否存在名为“斗罗大陆”的文件夹。
21. `pass`:如果存在,则不做任何操作;如果不存在,则创建名为“斗罗大陆”的文件夹。
22. `contents = re.findall('<div id="content">(.*?)</div>', response.text)`:使用正则表达式解析出章节内容。
23. `with open('./斗罗大陆/' + chapter_info['title'] + '.txt', 'w', encoding='utf-8') as f: ...`:以写入模式打开指定的文件,用于保存章节内容。
24. `for content in contents: ...`:遍历每一段内容。
25. `f.write(content.replace(' ', '').replace('<br/><br/>', '\n').strip())`:将内容写入文件,并将空格和换行符进行替换和清除。
26. `print('下载成功')`:在控制台输出下载成功的提示消息。
27. `if __name__ == '__main__':`:如果这个 Python 模块是作为主程序运行,则执行下面的代码。
28. `get_content(get_info(url))`:调用 get_info 函数,获取所有章节的信息,并将其作为参数传递给 get_content 函数,逐个下载每个章节的内容。
相关推荐
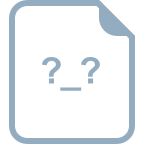
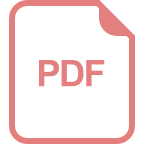
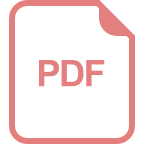















