1.Open input_a3.txt2.Read each expression and check if it’s a valid or not valid parentheses3.Write an output file “output_a3.txt”4.Calculate total execution of your programNote:Submit your program includes your source code*.java and output_a3.txt and READme.txtInput_a3.txt will be uploaded to your by tonight.用JAVA解答
时间: 2023-12-03 10:43:26 浏览: 90
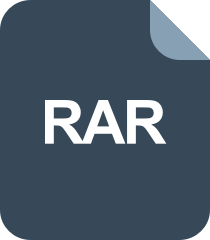
The-parentheses-matching-the-test.rar_Parentheses Matching_The T
以下是用 Java 实现的解答:
```java
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
import java.util.Stack;
public class ParenthesesChecker {
public static void main(String[] args) throws IOException {
// 读取输入文件名
Scanner scanner = new Scanner(System.in);
System.out.print("Enter input file name: ");
String inputFileName = scanner.nextLine();
// 读取输入文件
File inputFile = new File(inputFileName);
Scanner fileScanner = new Scanner(inputFile);
// 准备输出文件
String outputFileName = "output_a3.txt";
FileWriter fileWriter = new FileWriter(outputFileName);
// 逐行读取表达式并检查
long startTime = System.currentTimeMillis();
while (fileScanner.hasNextLine()) {
String expression = fileScanner.nextLine();
boolean isValid = checkParentheses(expression);
fileWriter.write(expression + " - " + (isValid ? "valid" : "not valid") + "\n");
}
long endTime = System.currentTimeMillis();
// 关闭文件和扫描器
fileWriter.close();
fileScanner.close();
// 输出执行时间
long executionTime = endTime - startTime;
System.out.println("Total execution time: " + executionTime + " ms");
}
private static boolean checkParentheses(String expression) {
Stack<Character> stack = new Stack<>();
for (int i = 0; i < expression.length(); i++) {
char ch = expression.charAt(i);
if (ch == '(' || ch == '{' || ch == '[') {
stack.push(ch);
} else if (ch == ')' || ch == '}' || ch == ']') {
if (stack.isEmpty()) {
return false;
}
char top = stack.pop();
if (ch == ')' && top != '(') {
return false;
} else if (ch == '}' && top != '{') {
return false;
} else if (ch == ']' && top != '[') {
return false;
}
}
}
return stack.isEmpty();
}
}
```
以上程序中,我们通过 `Scanner` 类读取用户输入的输入文件名,然后使用 `File` 类和 `Scanner` 类读取输入文件中的表达式。逐行读取后,我们调用 `checkParentheses()` 方法检查表达式是否括号匹配。如果是,我们就在输出文件中写入 "valid",否则写入 "not valid"。
`checkParentheses()` 方法中,我们使用 `Stack` 类来模拟栈,逐个遍历表达式中的字符。如果遇到左括号,我们就将其入栈。如果遇到右括号,我们就将栈顶的元素出栈,并检查它与当前右括号是否匹配。如果不匹配,我们就返回 `false`。如果表达式遍历完毕后栈非空,说明有左括号未被匹配,我们也返回 `false`。
最后,我们输出执行时间,即程序开始执行到输出结果之间所经过的时间。
需要注意的是,为了方便起见,我们在程序中没有对文件输入输出进行异常处理。在实际开发中,我们应该使用 `try-catch` 语句来处理可能出现的异常。
阅读全文
相关推荐
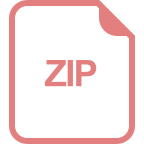















