用C语言编写程序:用射线法判断点在多边形内部,要求用户可以自定义点和多边形
时间: 2024-05-02 19:17:38 浏览: 9
以下是一个简单的用射线法判断点在多边形内部的C语言程序,可以让用户自定义点和多边形。
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_POINTS 100
typedef struct point {
float x;
float y;
} Point;
float cross_product(Point p1, Point p2) {
return p1.x * p2.y - p2.x * p1.y;
}
bool is_inside_polygon(Point point, Point polygon[], int num_points) {
int intersections = 0;
for (int i = 0; i < num_points; i++) {
Point p1 = polygon[i];
Point p2 = polygon[(i + 1) % num_points];
if ((point.y > p1.y && point.y <= p2.y) || (point.y > p2.y && point.y <= p1.y)) {
float x_intersection = (point.y - p1.y) * (p2.x - p1.x) / (p2.y - p1.y) + p1.x;
if (x_intersection < point.x) {
intersections++;
}
}
}
return intersections % 2 == 1;
}
int main() {
Point polygon[MAX_POINTS];
int num_points;
printf("Enter the number of points in the polygon: ");
scanf("%d", &num_points);
printf("Enter the points of the polygon:\n");
for (int i = 0; i < num_points; i++) {
printf("Point %d: ", i + 1);
scanf("%f %f", &polygon[i].x, &polygon[i].y);
}
Point point;
printf("Enter the point to test: ");
scanf("%f %f", &point.x, &point.y);
if (is_inside_polygon(point, polygon, num_points)) {
printf("Point is inside the polygon\n");
} else {
printf("Point is outside the polygon\n");
}
return 0;
}
```
程序中使用了射线法判断点是否在多边形内部。具体来说,对于点和多边形的每条边,如果点的纵坐标在边的两个端点的纵坐标之间,那么它与边的交点的横坐标可以通过线性插值计算得到,如果交点的横坐标小于点的横坐标,则说明射线与边有交点,交点数加一。如果交点数是奇数,则点在多边形内部,否则在外部。
相关推荐
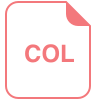
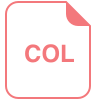
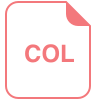
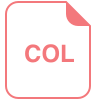
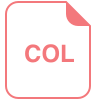









