ostream& operator<<(ostream& output,Complex& a){ output<<a.real<<"+"<<a.imag<<"i"<<endl; return output; }
时间: 2024-04-28 22:20:44 浏览: 40
这是一个重载了 << 运算符的函数,用于将 Complex 类型的对象以特定格式输出到流中。其中 ostream& 代表返回值为输出流的引用,Complex& a 代表传入的 Complex 类型的引用参数。该函数将实部和虚部输出到流中,并在虚部后添加了一个小写字母 i 表示虚数单位。最后返回输出流的引用。
相关问题
ostream & operator<<(ostream& cout,Person& p)
这是一个重载输出流运算符的函数,它的作用是将一个 Person 类型的对象输出到标准输出流中。具体实现方式可以参考以下代码:
```c++
#include<iostream>
#include<string>
using namespace std;
class Person{
public:
Person(string name,int age):m_name(name),m_age(age){}
friend ostream & operator<<(ostream& cout,Person& p);
private:
string m_name;
int m_age;
};
ostream & operator<<(ostream& cout,Person& p){
cout<<"Name: "<<p.m_name<<", Age: "<<p.m_age<<endl;
return cout;
}
int main(){
Person p("Tom", 18);
cout << p; // 输出 "Name: Tom, Age: 18"
return 0;
}
```
在上述代码中,重载输出流运算符的函数 `operator<<` 被定义为 `Person` 类的友元函数,它可以直接访问 `Person` 类的私有成员变量 `m_name` 和 `m_age`,并将它们输出到标准输出流中。在主函数中,我们创建一个 `Person` 对象 `p`,然后通过 `cout << p` 的方式将其输出到标准输出流中。
ostream &operator<<(ostream& out, Fraction& f)
这是一个C++中的运算符重载函数,它重载了插入运算符(<<),用于输出Fraction(分数)对象。
在这个函数中,第一个参数是一个输出流对象(通常是cout),第二个参数是Fraction对象的引用。这个函数返回一个输出流对象的引用,以便能够进行链式调用。
在函数中,可以使用引用参数中的分数对象的成员函数和数据成员,将分数对象的值输出到输出流对象中。例如,可以使用分数对象的分子和分母来输出分数的值。
示例代码:
```
ostream &operator<<(ostream& out, Fraction& f) {
out << f.getNumerator() << "/" << f.getDenominator();
return out;
}
```
这个函数将分数对象的值输出为分子/分母的形式。可以通过以下方式调用:
```
Fraction f(1, 2);
cout << f;
```
这将输出 1/2。
阅读全文
相关推荐
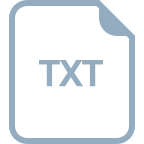
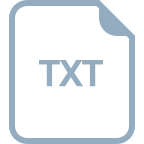
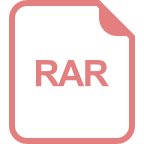













