用C++写一个散列表查找
时间: 2023-03-24 07:03:23 浏览: 104
好的,我可以回答这个问题。散列表是一种常用的数据结构,可以快速地查找和插入数据。下面是一个用 C 语言实现的散列表查找的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 100
typedef struct {
char* key;
char* value;
} Entry;
typedef struct {
Entry* entries[TABLE_SIZE];
} HashTable;
unsigned int hash(const char* key) {
unsigned int hash = ;
for (int i = ; i < strlen(key); i++) {
hash = hash * 31 + key[i];
}
return hash % TABLE_SIZE;
}
void insert(HashTable* table, const char* key, const char* value) {
unsigned int index = hash(key);
Entry* entry = malloc(sizeof(Entry));
entry->key = strdup(key);
entry->value = strdup(value);
table->entries[index] = entry;
}
char* lookup(HashTable* table, const char* key) {
unsigned int index = hash(key);
if (table->entries[index] != NULL && strcmp(table->entries[index]->key, key) == ) {
return table->entries[index]->value;
} else {
return NULL;
}
}
int main() {
HashTable table;
insert(&table, "apple", "a fruit");
insert(&table, "banana", "another fruit");
insert(&table, "carrot", "a vegetable");
char* value = lookup(&table, "apple");
if (value != NULL) {
printf("The value of 'apple' is '%s'\n", value);
} else {
printf("Key not found\n");
}
value = lookup(&table, "orange");
if (value != NULL) {
printf("The value of 'orange' is '%s'\n", value);
} else {
printf("Key not found\n");
}
return ;
}
```
这个散列表使用了字符串作为键和值,使用了简单的哈希函数来计算键的哈希值,并使用链表解决哈希冲突。你可以根据需要修改哈希函数和解决哈希冲突的方法。
相关推荐
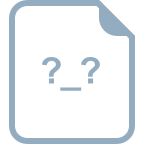














