写一个带 AABB的Octree算法的C++实现
时间: 2023-05-20 19:00:47 浏览: 51
非常感谢您的提问。以下是一个简单的带 AABB 的 Octree 算法的 C 实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_DEPTH 8
typedef struct {
float x, y, z;
} Vector3;
typedef struct {
Vector3 min, max;
} AABB;
typedef struct OctreeNode OctreeNode;
struct OctreeNode {
AABB bounds;
OctreeNode* children[8];
};
OctreeNode* createOctreeNode(AABB bounds, int depth) {
OctreeNode* node = (OctreeNode*)malloc(sizeof(OctreeNode));
node->bounds = bounds;
for (int i = 0; i < 8; i++) {
node->children[i] = NULL;
}
return node;
}
void insertOctreeNode(OctreeNode* node, AABB bounds, int depth) {
if (depth >= MAX_DEPTH) {
return;
}
Vector3 center = {
(bounds.min.x + bounds.max.x) / 2,
(bounds.min.y + bounds.max.y) / 2,
(bounds.min.z + bounds.max.z) / 2
};
int index = 0;
if (bounds.min.x > center.x) index |= 1;
if (bounds.min.y > center.y) index |= 2;
if (bounds.min.z > center.z) index |= 4;
if (node->children[index] == NULL) {
AABB childBounds = {
{ center.x, center.y, center.z },
{ bounds.max.x, bounds.max.y, bounds.max.z }
};
node->children[index] = createOctreeNode(childBounds, depth + 1);
}
insertOctreeNode(node->children[index], bounds, depth + 1);
}
void printOctreeNode(OctreeNode* node) {
printf("Bounds: (%f, %f, %f) - (%f, %f, %f)\n", node->bounds.min.x, node->bounds.min.y, node->bounds.min.z, node->bounds.max.x, node->bounds.max.y, node->bounds.max.z);
for (int i = 0; i < 8; i++) {
if (node->children[i] != NULL) {
printf("Child %d:\n", i);
printOctreeNode(node->children[i]);
}
}
}
int main() {
AABB rootBounds = {
{ -1, -1, -1 },
{ 1, 1, 1 }
};
OctreeNode* rootNode = createOctreeNode(rootBounds, 0);
AABB bounds1 = {
{ -0.5, -0.5, -0.5 },
{ 0.5, 0.5, 0.5 }
};
insertOctreeNode(rootNode, bounds1, 0);
AABB bounds2 = {
{ 0.5, 0.5, 0.5 },
{ 1, 1, 1 }
};
insertOctreeNode(rootNode, bounds2, 0);
printOctreeNode(rootNode);
return 0;
}
```
希望这个实现能够帮助您理解带 AABB 的 Octree 算法。如果您有任何问题或建议,请随时告诉我。
相关推荐
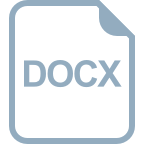
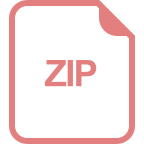














