用大写字母I表示入栈操作,用大写字母O表示出栈操作,针对由I和O组成的序列,判断其操作是否正确可行。其错误操作可能有I入栈时栈满、O出栈时栈空,最后序列检查结束栈不为空,第一行和第二行输入两个正整数n、m,其中n为代测序列的个数,m为堆栈的最大容量,接下来n行每行输入一个仅由I和O构成的序列,序列非空且长度不超过100,针对每个输入序列,检查该序列的正确性,如果正确则输出yes,错误则输出no,用c语言代码实现
时间: 2023-06-04 13:04:12 浏览: 111
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct {
int top;
int capacity;
char* data;
} Stack;
Stack* createStack(int capacity) {
Stack* stack = (Stack*) malloc(sizeof(Stack));
if (!stack) {
return NULL;
}
stack->top = -1;
stack->capacity = capacity;
stack->data = (char*) malloc(capacity * sizeof(char));
if (!stack->data) {
return NULL;
}
return stack;
}
void destroyStack(Stack* stack) {
if (stack) {
if (stack->data) {
free(stack->data);
}
free(stack);
}
}
int isFull(Stack* stack) {
return stack->top == stack->capacity - 1;
}
int isEmpty(Stack* stack) {
return stack->top == -1;
}
char top(Stack* stack) {
return stack->data[stack->top];
}
void push(Stack* stack, char element) {
if (isFull(stack)) {
return;
}
stack->data[++stack->top] = element;
}
char pop(Stack* stack) {
if (isEmpty(stack)) {
return '\0';
}
return stack->data[stack->top--];
}
void check(char* sequence, int capacity) {
Stack* stack = createStack(capacity);
int i = 0;
while (sequence[i] != '\0') {
if (sequence[i] == 'I') {
if (isFull(stack)) {
printf("no\n");
destroyStack(stack);
return;
}
push(stack, 'I');
} else if (sequence[i] == 'O') {
if (isEmpty(stack)) {
printf("no\n");
destroyStack(stack);
return;
}
pop(stack);
}
i++;
}
if (!isEmpty(stack)) {
printf("no\n");
destroyStack(stack);
return;
}
printf("yes\n");
destroyStack(stack);
}
int main() {
int n, capacity;
scanf("%d %d\n", &n, &capacity);
char sequence[MAX_SIZE + 1];
for (int i = 0; i < n; i++) {
fgets(sequence, MAX_SIZE + 1, stdin);
sequence[strcspn(sequence, "\n")] = '\0'; // 去掉换行符
check(sequence, capacity);
}
return 0;
}
```
阅读全文
相关推荐
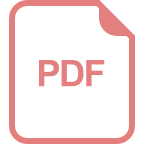
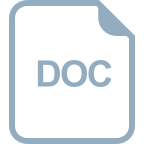

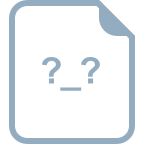
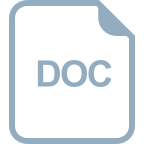
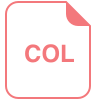



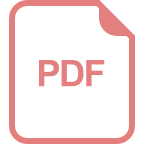
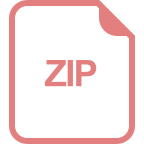
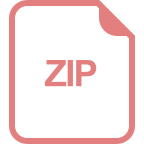
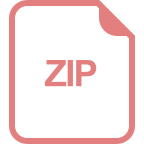
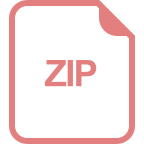
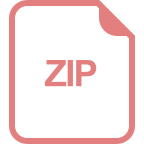