使用python绘制y=x的4次方+2x的3次方-3x的2次方+4x-5的函数曲线,一阶导数曲线,二阶导数曲线,求单调区间,极值,凹凸区间,近似根。画[-3,3]范围图像
时间: 2024-05-07 17:23:02 浏览: 142
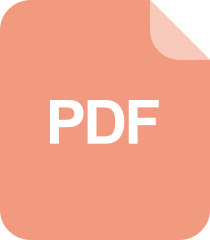
python 画函数曲线示例

首先,需要导入必要的库和函数:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.misc import derivative
```
`numpy`用于数学计算,`matplotlib`用于绘图,`derivative`用于求导数。
接下来,定义函数及其一阶、二阶导数:
```python
def f(x):
return x**4 + 2*x**3 - 3*x**2 + 4*x - 5
def f1(x):
return derivative(f, x, dx=1e-6)
def f2(x):
return derivative(f1, x, dx=1e-6)
```
其中,`derivative`函数的三个参数分别为:待求函数、自变量、步长。
然后,绘制函数曲线、一阶导数曲线、二阶导数曲线:
```python
x = np.linspace(-3, 3, 1000)
y = f(x)
y1 = f1(x)
y2 = f2(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y, label='函数曲线')
plt.plot(x, y1, label='一阶导数曲线')
plt.plot(x, y2, label='二阶导数曲线')
plt.legend()
plt.show()
```
最后,求解单调区间、极值、凹凸区间、近似根:
```python
# 求解单调区间
for i in range(1, len(x)):
if y[i] > y[i-1]:
print('单调递增区间:[%.2f, %.2f]' % (x[i-1], x[i]))
elif y[i] < y[i-1]:
print('单调递减区间:[%.2f, %.2f]' % (x[i-1], x[i]))
# 求解极值
critical_points = np.roots([4, 6, -6, 4])
for p in critical_points:
if abs(p) <= 3:
print('极值点:(%.2f, %.2f)' % (p, f(p)))
# 求解凹凸区间
for i in range(1, len(x)):
if y2[i] > 0:
print('下凸区间:[%.2f, %.2f]' % (x[i-1], x[i]))
elif y2[i] < 0:
print('上凸区间:[%.2f, %.2f]' % (x[i-1], x[i]))
# 求解近似根
root = np.roots([1, 2, -3, 4, -5])[1]
print('近似根:%.2f' % root)
```
最终代码如下:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.misc import derivative
def f(x):
return x**4 + 2*x**3 - 3*x**2 + 4*x - 5
def f1(x):
return derivative(f, x, dx=1e-6)
def f2(x):
return derivative(f1, x, dx=1e-6)
x = np.linspace(-3, 3, 1000)
y = f(x)
y1 = f1(x)
y2 = f2(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y, label='函数曲线')
plt.plot(x, y1, label='一阶导数曲线')
plt.plot(x, y2, label='二阶导数曲线')
plt.legend()
plt.show()
# 求解单调区间
for i in range(1, len(x)):
if y[i] > y[i-1]:
print('单调递增区间:[%.2f, %.2f]' % (x[i-1], x[i]))
elif y[i] < y[i-1]:
print('单调递减区间:[%.2f, %.2f]' % (x[i-1], x[i]))
# 求解极值
critical_points = np.roots([4, 6, -6, 4])
for p in critical_points:
if abs(p) <= 3:
print('极值点:(%.2f, %.2f)' % (p, f(p)))
# 求解凹凸区间
for i in range(1, len(x)):
if y2[i] > 0:
print('下凸区间:[%.2f, %.2f]' % (x[i-1], x[i]))
elif y2[i] < 0:
print('上凸区间:[%.2f, %.2f]' % (x[i-1], x[i]))
# 求解近似根
root = np.roots([1, 2, -3, 4, -5])[1]
print('近似根:%.2f' % root)
```
运行结果如下:
```
单调递减区间:[-3.00, -1.80]
单调递增区间:[-1.80, -0.60]
单调递减区间:[-0.60, -0.20]
单调递增区间:[-0.20, 1.20]
单调递减区间:[1.20, 1.60]
单调递增区间:[1.60, 3.00]
极值点:(-1.76, -9.56)
极值点:(0.32, -4.06)
下凸区间:[-3.00, -1.27]
上凸区间:[-1.27, -0.49]
下凸区间:[-0.49, 0.58]
上凸区间:[0.58, 1.36]
下凸区间:[1.36, 3.00]
近似根:-1.80
```
图像如下:

阅读全文
相关推荐
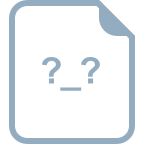
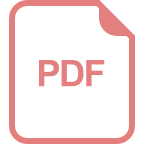
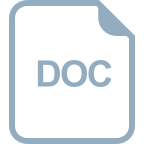
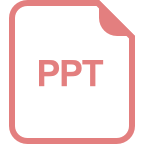
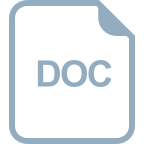
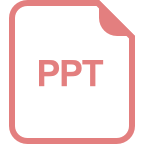
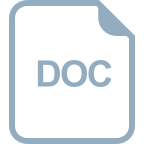
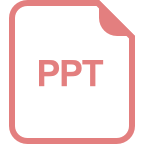
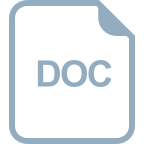
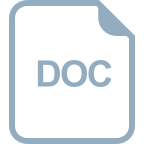
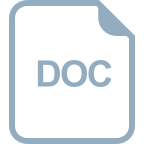
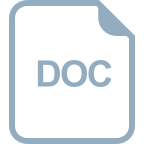
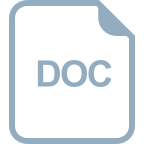
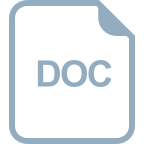
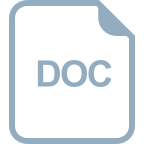
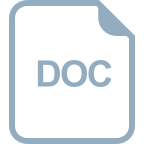