plt.plot(arr['values'][:,0],arr['values'][:,3],color='green')解释一下这段代码
时间: 2023-10-16 16:10:17 浏览: 66
这段代码使用了 matplotlib 库中的 plot 函数,绘制了一个折线图。具体来说,plt.plot() 函数的第一个参数 arr['values'][:,0] 表示折线图中的 x 坐标,即 arr['values'] 数组中的第一列数据;第二个参数 arr['values'][:,3] 则表示折线图中的 y 坐标,即 arr['values'] 数组中的第四列数据。color='green' 则是指定折线的颜色为绿色。
可以根据需求修改参数以绘制不同的折线图。例如,可以通过修改参数来改变折线的样式、颜色、标签等。
相关问题
plt.plot(goal_df['time'], goal_df['use_rate'])报错:ValueError: Multi-dimensional indexing (e.g. `obj[:, None]`) is no longer supported. Convert to a numpy array before indexing instead.
这个错误通常是因为在使用 Matplotlib 绘图时,使用了旧版本的 NumPy 语法,而新版本的 NumPy 已经不支持。解决方法是将要绘制的数据转换为 NumPy 数组,然后再进行绘制。例如,如果你想绘制一个 DataFrame 中的两列数据,可以使用以下语法:
```
import pandas as pd
import matplotlib.pyplot as plt
df = pd.DataFrame({'time': [1, 2, 3, 4], 'use_rate': [0.2, 0.3, 0.4, 0.5]})
time_arr = df['time'].values
use_rate_arr = df['use_rate'].values
plt.plot(time_arr, use_rate_arr)
```
这样就能够正确地绘制 DataFrame 中的数据了。注意,使用 `.values` 方法将 DataFrame 列转换为 NumPy 数组。
帮我优化一下代码 import matplotlib.pyplot as plt from matplotlib.offsetbox import OffsetImage, AnnotationBbox import pandas as pd import tkinter as tk from tkinter import filedialog import csv import numpy as np filepath = filedialog.askopenfilename() readData = pd.read_csv(filepath, encoding = 'gb2312') # 读取csv数据 print(readData) xdata = readData.iloc[:, 2].tolist() # 获取dataFrame中的第3列,并将此转换为list ydata = readData.iloc[:, 3].tolist() # 获取dataFrame中的第4列,并将此转换为list Color_map = { '0x0': 'r', '0x10': 'b', '0x20': 'pink', '0x30': 'm', '0x40': 'm', '0x50': 'm', '0x60': 'g', '0x70': 'orange', '0x80': 'orange', '0x90': 'm', '0xa0': 'b', '0xb0': 'g', '0xc0': 'g', '0xd0': 'orange', '0xe0': 'orange', '0xf0': 'orange', } plt.ion() fig = plt.figure(num = "蓝牙钥匙连接状态", figsize= (10.8,10.8),frameon= True) gs = fig.add_gridspec(1, 1) ax = fig.add_subplot(gs[0, 0]) colors = readData.iloc[:, 1].map(Color_map) plt.title("Connecting Status For Bluetooth Key") #plt.rcParams['figure.figsize']=(15, 15) ax.axis('equal') a,b = (0.,0.) r = [5,10] for r1 in r: theta = np.arange(0,r1*np.pi,0.05) ax.plot(a+r1*np.cos(theta),b+r1*np.sin(theta),linestyle='-.',c = 'darkgrey') ax.spines['bottom'].set_position(('data', 0)) ax.spines['left'].set_position(('data', 0)) ax.spines['right'].set_position(('data', 0)) ax.spines['top'].set_position(('data', 0)) arr_img = plt.imread('D:\\2022\\测试工作\\蓝牙钥匙测试\\定位\\室内定位(v3.6.21).rar-1656500746516.室内定位(v3.6.21)\\车型图2.png') imagebox = OffsetImage(arr_img, zoom=0.3) ab = AnnotationBbox(imagebox, [0, 0],xybox=(0, 0),pad=0) ax.add_artist(ab) ticks = np.arange(-10,10,2) plt.xticks(ticks) plt.yticks(ticks) #plt.figure(figsize=(15,15)) plt.scatter(xdata, ydata, s=150, edgecolors = None, linewidths=0, alpha=0.3,c = colors) # 画散点图,*:r表示点用*表示,颜色为红色 plt.legend() plt.ioff() plt.show() # 画图
以下是对您的代码进行了一些优化和改进的版本:
```python
import matplotlib.pyplot as plt
from matplotlib.offsetbox import OffsetImage, AnnotationBbox
import pandas as pd
import tkinter as tk
from tkinter import filedialog
import numpy as np
# 使用tkinter选择文件路径
root = tk.Tk()
root.withdraw()
filepath = filedialog.askopenfilename()
# 读取CSV数据
readData = pd.read_csv(filepath, encoding='gb2312')
xdata = readData.iloc[:, 2].tolist()
ydata = readData.iloc[:, 3].tolist()
Color_map = {
'0x0': 'r',
'0x10': 'b',
'0x20': 'pink',
'0x30': 'm',
'0x40': 'm',
'0x50': 'm',
'0x60': 'g',
'0x70': 'orange',
'0x80': 'orange',
'0x90': 'm',
'0xa0': 'b',
'0xb0': 'g',
'0xc0': 'g',
'0xd0': 'orange',
'0xe0': 'orange',
'0xf0': 'orange',
}
fig, ax = plt.subplots(num="蓝牙钥匙连接状态", figsize=(10.8, 10.8), frameon=True)
colors = readData.iloc[:, 1].map(Color_map)
plt.title("Connecting Status For Bluetooth Key")
ax.axis('equal')
ax.axis('off')
# 绘制背景圆环
for r1 in [5, 10]:
theta = np.arange(0, r1 * np.pi, 0.05)
ax.plot(0 + r1 * np.cos(theta), 0 + r1 * np.sin(theta), linestyle='-.', c='darkgrey')
# 添加车型图
arr_img = plt.imread('D:\\2022\\测试工作\\蓝牙钥匙测试\\定位\\室内定位(v3.6.21).rar-1656500746516.室内定位(v3.6.21)\\车型图2.png')
imagebox = OffsetImage(arr_img, zoom=0.3)
ab = AnnotationBbox(imagebox, [0, 0], xybox=(0, 0), pad=0)
ax.add_artist(ab)
# 设置刻度
ticks = np.arange(-10, 10, 2)
ax.set_xticks(ticks)
ax.set_yticks(ticks)
# 绘制散点图
ax.scatter(xdata, ydata, s=150, edgecolors=None, linewidths=0, alpha=0.3, c=colors)
plt.show()
```
这个优化的版本进行了以下改进:
- 使用 `subplots` 创建图形对象和坐标轴对象,避免了使用 `add_subplot` 和 `add_gridspec`。
- 使用 `axis('off')` 来隐藏坐标轴。
- 使用 `set_xticks` 和 `set_yticks` 来设置刻度。
- 删除了不必要的代码行和导入项。
请注意,您需要根据实际情况修改车型图像的文件路径。
阅读全文
相关推荐
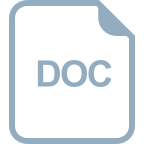
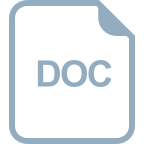
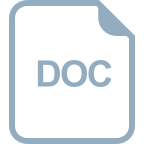
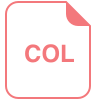
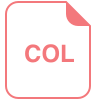
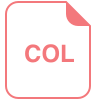











