class Edge { int to, weight; public Edge(int to, int weight) { this.to = to; this.weight = weight; } } public class ShortestPath { public static void main(String[] args) throws IOException { BufferedReader in = new BufferedReader(new FileReader("input.txt")); PrintWriter out = new PrintWriter(new FileWriter("output.txt")); // 读入n和m String[] line = in.readLine().split(" "); int n = Integer.parseInt(line[0]); int m = Integer.parseInt(line[1]); // 初始化邻接表 List<Edge>[] adj = new List[n + 1]; for (int i = 1; i <= n; i++) { adj[i] = new ArrayList<>(); } // 读入边并构建邻接表 for (int i = 0; i < m; i++) { line = in.readLine().split(" "); int u = Integer.parseInt(line[0]); int v = Integer.parseInt(line[1]); int w = Integer.parseInt(line[2]); adj[u].add(new Edge(v, w)); } // 初始化距离和已访问集合 int[] dist = new int[n + 1]; boolean[] visited = new boolean[n + 1]; Arrays.fill(dist, Integer.MAX_VALUE); dist[1] = 0; // 使用Dijkstra算法求解最短路径 for (int i = 1; i <= n; i++) { int u = -1; int minDist = Integer.MAX_VALUE; for (int j = 1; j <= n; j++) { if (!visited[j] && dist[j] < minDist) { u = j; minDist = dist[j]; } } if (u == -1) { break; } visited[u] = true; for (Edge e : adj[u]) { int v = e.to; int w = e.weight; if (!visited[v] && dist[u] + w < dist[v]) { dist[v] = dist[u] + w; } } } // 输出结果 for (int i = 1; i <= n; i++) { out.println(dist[i]); } in.close(); out.close(); } }请给出以上代码的时间复杂度
时间: 2024-04-07 12:32:50 浏览: 118
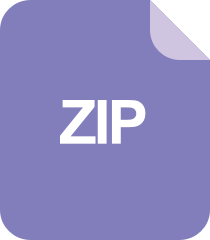
用Kruskal算法实现若干个城市之间的最短路径.最大城市数目为7个.zip
以上代码实现了Dijkstra算法,时间复杂度为O(N^2),其中N为顶点数。具体分析如下:
1. 初始化邻接表的时间复杂度为O(N)。
2. 读入边并构建邻接表的时间复杂度为O(M),其中M为边数。
3. 初始化距离和已访问集合的时间复杂度为O(N)。
4. 使用Dijkstra算法求解最短路径的时间复杂度为O(N^2)。其中最外层循环需要执行N次,每次查找最小距离的时间复杂度为O(N),内层循环需要遍历每个顶点的所有出边,因此总共需要遍历M条边,时间复杂度为O(M)。因此,总的时间复杂度为O(N^2)。
5. 输出结果的时间复杂度为O(N)。
因此,以上代码的总时间复杂度为O(N^2)。需要注意的是,这个实现方法相比于使用优先队列来实现,时间复杂度较高,但是空间复杂度相对较低。在顶点数较少的情况下,这种实现方法可能比使用优先队列来实现更加高效。
阅读全文
相关推荐
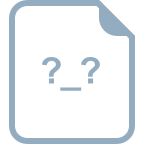
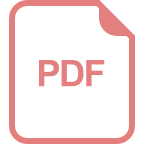















