ros用c++写个串口通信代码
时间: 2023-03-20 19:03:43 浏览: 130
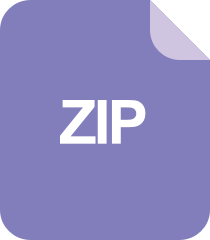
计算机和PLC进行串口通信 源代码 C++
可以使用ROS提供的serial包来实现串口通信。以下是使用C语言编写的一个简单示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios opt;
// 打开串口设备文件
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 设置串口属性
tcgetattr(fd, &opt);
cfsetispeed(&opt, B9600);
cfsetospeed(&opt, B9600);
opt.c_cflag &= ~PARENB;
opt.c_cflag &= ~CSTOPB;
opt.c_cflag &= ~CSIZE;
opt.c_cflag |= CS8;
opt.c_cflag &= ~CRTSCTS;
opt.c_cflag |= CREAD | CLOCAL;
opt.c_iflag &= ~(IXON | IXOFF | IXANY);
opt.c_iflag &= ~(ICANON | ECHO | ECHOE | ISIG);
opt.c_oflag &= ~OPOST;
opt.c_cc[VMIN] = 1;
opt.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &opt);
// 读取串口数据
char buf[255];
while (1) {
int n = read(fd, buf, 255);
if (n > 0) {
buf[n] = '\0';
printf("Received: %s", buf);
}
}
return 0;
}
```
该代码使用了POSIX标准的termios库来配置串口属性,并通过read函数读取串口数据。可以根据实际需要修改串口设备文件名、波特率和其他属性。
阅读全文
相关推荐


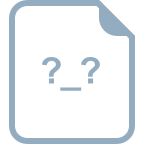



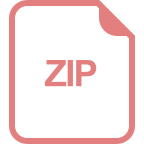
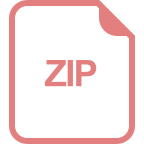
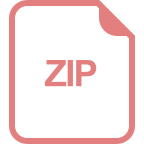
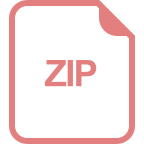
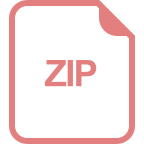
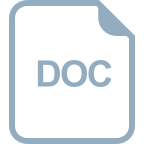
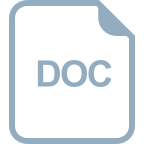


