用c++编写飞机大战代码
时间: 2024-06-08 10:05:30 浏览: 8
以下是一个简单的飞机大战游戏的 C++ 代码示例:
```c++
#include <iostream>
#include <windows.h>
#include <conio.h>
using namespace std;
const int width = 40; // 游戏区域宽度
const int height = 20; // 游戏区域高度
int x, y; // 玩家飞机坐标
int bx, by; // 子弹坐标
int ex, ey; // 敌机坐标
int score; // 分数
void setCursor(bool visible) {
HANDLE console = GetStdHandle(STD_OUTPUT_HANDLE);
CONSOLE_CURSOR_INFO lpCursor;
lpCursor.bVisible = visible;
lpCursor.dwSize = visible ? 20 : 1;
SetConsoleCursorInfo(console, &lpCursor);
}
void gotoxy(int x, int y) {
HANDLE console = GetStdHandle(STD_OUTPUT_HANDLE);
COORD cursor = { x, y };
SetConsoleCursorPosition(console, cursor);
}
void drawBorder() {
for (int i = 0; i < width + 2; i++) cout << "#";
cout << endl;
for (int i = 0; i < height; i++) {
for (int j = 0; j < width + 2; j++) {
if (j == 0 || j == width + 1) cout << "#";
else cout << " ";
}
cout << endl;
}
for (int i = 0; i < width + 2; i++) cout << "#";
cout << endl;
}
void drawPlayer() {
gotoxy(x, y); cout << "^";
}
void drawBullet() {
gotoxy(bx, by); cout << "|";
}
void drawEnemy() {
gotoxy(ex, ey); cout << "V";
}
void updateBullet() {
if (by > 0) {
drawBullet();
by--;
} else {
bx = 0;
by = 0;
}
}
void updateEnemy() {
drawEnemy();
ey++;
if (ey == height) {
ex = rand() % width + 1;
ey = 1;
score++;
}
}
void updateScore() {
gotoxy(0, height + 1);
cout << "Score: " << score;
}
void gameOver() {
system("cls");
setCursor(true);
gotoxy(width / 2 - 5, height / 2);
cout << "GAME OVER" << endl;
gotoxy(width / 2 - 7, height / 2 + 1);
cout << "Your score: " << score << endl;
exit(0);
}
int main() {
setCursor(false);
srand(time(NULL));
x = width / 2;
y = height - 1;
bx = 0;
by = 0;
ex = rand() % width + 1;
ey = 1;
score = 0;
drawBorder();
drawPlayer();
while (true) {
if (_kbhit()) {
switch (_getch()) {
case 'a':
if (x > 1) x--;
break;
case 'd':
if (x < width) x++;
break;
case ' ':
if (by == 0) {
bx = x;
by = y - 1;
}
break;
case 'q':
exit(0);
break;
}
}
updateBullet();
updateEnemy();
if (bx == ex && by == ey) {
score++;
ex = rand() % width + 1;
ey = 1;
bx = 0;
by = 0;
}
if ((x == ex && y == ey) || (x == ex - 1 && y == ey)) {
gameOver();
}
drawBorder();
drawPlayer();
updateScore();
Sleep(50);
}
return 0;
}
```
这是一个非常简单的示例,只实现了玩家移动、发射子弹、敌机下落和碰撞检测等基本功能。在实际开发中,还需要添加更多的游戏元素和功能。
相关推荐
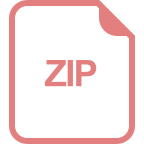














