使用python建立一个三维装箱问题
时间: 2023-11-25 17:07:19 浏览: 152
三维装箱问题是一个经典的组合优化问题,它的目标是在给定的一批物品中,将它们装入尽可能少的箱子中,使得每个箱子的总体积不超过限制。这个问题可以用Python来求解。下面是一个简单的实现示例:
```python
from typing import List, Tuple
def pack_boxes(items: List[Tuple[int, int, int]], max_volume: int) -> int:
"""Pack items into boxes with maximum volume"""
items = sorted(items, key=lambda x: x[0]*x[1]*x[2], reverse=True)
boxes = []
for item in items:
packed = False
for box in boxes:
if box[3] + item[0]*item[1]*item[2] <= max_volume:
box[0].append(item)
box[1] += item[0]
box[2] += item[1]
box[3] += item[2]
packed = True
break
if not packed:
boxes.append(([item], item[0], item[1], item[2]))
return len(boxes)
```
这个函数接受一个物品列表 `items` 和一个最大体积限制 `max_volume`,并返回需要的箱子数量。每个物品表示为一个元组 `(length, width, height)`。算法首先按照物品体积从大到小排序,然后从大到小地尝试将它们放入已有的箱子中,如果无法放入,则新建一个箱子。在放入物品时,算法记录箱子的长度、宽度和高度,以及已经使用的体积。
这个算法的时间复杂度为 $O(n^2)$,其中 $n$ 是物品数量。在实际应用中,可能需要使用更高效的算法,例如分支界限或遗传算法。
阅读全文
相关推荐
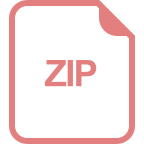
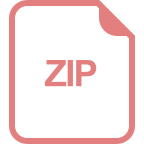
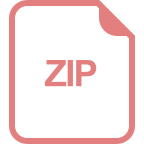

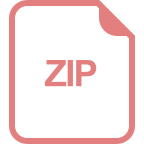













