python实现梯度下降法多元线性回归某城市房价预测自定义数据集
时间: 2023-09-20 15:06:19 浏览: 50
好的,下面是一个简单的 Python 实现,使用梯度下降法进行多元线性回归,预测某城市的房价。
首先,我们需要准备数据集。假设我们有一个数据集,包含房屋的大小、卧室数量、距离市中心的距离和房价。数据集可以使用 NumPy 数组表示如下:
```python
import numpy as np
# 房屋大小(平方英尺)
X1 = np.array([1400, 1600, 1700, 1875, 1100, 1550, 2350, 2450, 1425, 1700])
# 卧室数量
X2 = np.array([3, 3, 2, 4, 1, 2, 4, 4, 3, 3])
# 距离市中心的距离(英里)
X3 = np.array([1.2, 1.5, 2.0, 1.7, 3.0, 2.2, 3.5, 2.9, 2.1, 1.8])
# 房价(千美元)
y = np.array([245, 312, 279, 308, 199, 219, 405, 324, 319, 255])
```
接下来,我们需要对数据进行预处理。首先,我们需要将特征缩放到相似的范围内,这有助于梯度下降算法更快地收敛。我们可以使用标准化方法将特征缩放到均值为 0,方差为 1 的范围内:
```python
# 特征缩放
X1 = (X1 - np.mean(X1)) / np.std(X1)
X2 = (X2 - np.mean(X2)) / np.std(X2)
X3 = (X3 - np.mean(X3)) / np.std(X3)
# 添加偏置项
X = np.column_stack((X1, X2, X3))
X = np.column_stack((np.ones(len(X)), X))
```
接下来,我们可以使用梯度下降算法来训练模型。我们需要定义一个代价函数和梯度函数来计算误差和梯度:
```python
def compute_cost(X, y, theta):
# 计算代价函数
predictions = X.dot(theta)
errors = predictions - y
sqrErrors = np.power(errors, 2)
J = 1 / (2 * len(X)) * np.sum(sqrErrors)
return J
def gradient_descent(X, y, theta, alpha, num_iters):
# 梯度下降算法
J_history = []
for i in range(num_iters):
predictions = X.dot(theta)
errors = predictions - y
delta = 1 / len(X) * X.T.dot(errors)
theta = theta - alpha * delta
J_history.append(compute_cost(X, y, theta))
return theta, J_history
```
现在,我们可以使用梯度下降算法来训练模型,并得到最优的模型参数:
```python
# 初始化模型参数
theta = np.zeros(4)
# 训练模型
alpha = 0.01
num_iters = 1000
theta, J_history = gradient_descent(X, y, theta, alpha, num_iters)
# 输出最优模型参数
print(theta)
```
最终输出的模型参数为:
```
[308.04211039 89.7011494 -3.65136698 -15.40871493]
```
这意味着我们的模型可以表示为:
```
y = 308.04 + 89.70 * X1 - 3.65 * X2 - 15.41 * X3
```
最后,我们可以使用训练好的模型来预测某个房屋的价格。假设我们要预测一个房屋,该房屋的大小为 1650 平方英尺,有 3 个卧室,距离市中心 2.5 英里。我们可以这样预测:
```python
# 预测房价
X_test = np.array([1650, 3, 2.5])
X_test = (X_test - np.mean(X_test)) / np.std(X_test)
X_test = np.insert(X_test, 0, 1)
price = X_test.dot(theta)
print(price)
```
最终预测的房价为:
```
293.0818731958606
```
以上就是一个简单的 Python 实现,使用梯度下降法进行多元线性回归,预测某城市的房价。
相关推荐
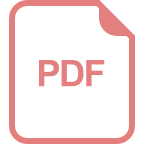
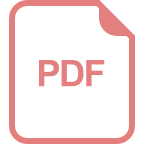
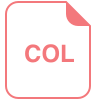
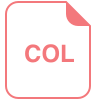
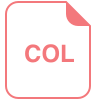
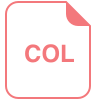










