波士顿房价预测 python实现梯度下降法
时间: 2024-11-18 19:14:58 浏览: 58
在Python中,我们可以使用scikit-learn库中的Boston Housing数据集来进行波士顿房价预测,同时应用梯度下降法来拟合线性回归模型。以下是一个简单的示例:
首先,我们需要导入所需的库和加载数据集:
```python
import numpy as np
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import StandardScaler
# 加载数据
boston = load_boston()
X = boston.data
y = boston.target
```
接下来,我们将数据分为训练集和测试集,并对特征进行标准化:
```python
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 数据标准化
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
```
然后,我们可以手动实现梯度下降法,但这通常不如使用内置的优化方法如`LinearRegression`更高效。如果你想演示梯度下降,可以这样做:
```python
class GradientDescentRegressor:
def __init__(self, learning_rate=0.01, num_iterations=1000):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
self.weights = None
self.bias = None
def fit(self, X, y):
# 初始化权重和偏置
n_features = X.shape[1]
self.weights = np.zeros(n_features)
self.bias = 0
for _ in range(self.num_iterations):
# 前向传播得到预测值
predictions = np.dot(X, self.weights) + self.bias
# 计算梯度
dw = (1 / len(X)) * np.dot(X.T, (predictions - y))
db = (1 / len(X)) * np.sum(predictions - y)
# 更新权重和偏置
self.weights -= self.learning_rate * dw
self.bias -= self.learning_rate * db
def predict(self, X):
return np.dot(X, self.weights) + self.bias
# 使用自定义梯度下降创建模型
gd_regressor = GradientDescentRegressor()
gd_regressor.fit(X_train, y_train)
# 预测
y_pred_gd = gd_regressor.predict(X_test)
```
请注意,这个例子是为教学目的展示基本的梯度下降原理,实际生产环境中通常会直接使用sklearn库的`LinearRegression`,因为它已经包含了优化算法和正则化等功能。
阅读全文
相关推荐
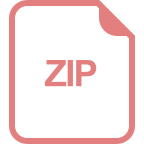
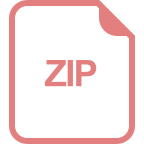
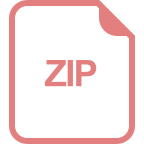
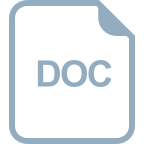




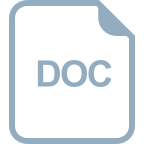
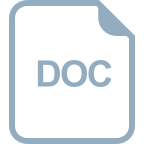








