在Linux系统下写一个C语言程序,用户可以自己输入一段格式为:$GNRMC,<1>,<2>,<3>,<4>,<5>,<6>,<7>,<8>,<9>,,,<11>,<12>*hh<CR><LF>的数据,程序通过数逗号的方式解析出数据中的日期和年月日信息,<1>是UTC时间,hhmmss.sss格式,<9>是UTC日期,DDMMYY格式,例如:$GNRMC,060723.000,A2236.91110,N11400.25548,E0.00,0.00,110623,,,A,V*69,数据中时间为2023年6月11日6时7分23秒,读取到以后将日期和时间转换为北京时间和日期并输入。
时间: 2024-03-25 22:40:30 浏览: 17
好的,这是一个比较具体的问题,下面是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_DATA_LEN 256
int main() {
char data[MAX_DATA_LEN];
printf("请输入GPS数据:");
fgets(data, MAX_DATA_LEN, stdin);
// 解析时间和日期
char *ptr = strtok(data, ",");
int hour = atoi(ptr + 2); // UTC时间的时
int minute = atoi(ptr + 4); // UTC时间的分
int second = atoi(ptr + 6); // UTC时间的秒
ptr = strtok(NULL, ",");
char *date_str = ptr + 4; // UTC日期的字符串
int day = atoi(date_str);
int month = atoi(date_str + 2);
int year = 2000 + atoi(date_str + 4);
// 转换为北京时间和日期
time_t utc_time;
struct tm *utc_tm;
utc_tm = (struct tm *) malloc(sizeof(struct tm));
utc_tm->tm_year = year - 1900;
utc_tm->tm_mon = month - 1;
utc_tm->tm_mday = day;
utc_tm->tm_hour = hour;
utc_tm->tm_min = minute;
utc_tm->tm_sec = second;
utc_time = mktime(utc_tm) + 8 * 3600; // 转换为北京时间
struct tm *bj_tm;
bj_tm = localtime(&utc_time);
// 输出北京时间和日期
printf("北京时间:%04d-%02d-%02d %02d:%02d:%02d\n",
bj_tm->tm_year + 1900, bj_tm->tm_mon + 1, bj_tm->tm_mday,
bj_tm->tm_hour, bj_tm->tm_min, bj_tm->tm_sec);
return 0;
}
```
这个程序通过 `fgets` 函数读取用户输入的 GPS 数据,然后使用 `strtok` 函数解析出其中的时间和日期信息。接着,使用 `mktime` 函数将 UTC 时间转换为 time_t 类型的时间戳,再使用 `localtime` 函数将时间戳转换为 struct tm 类型的北京时间。最后,将北京时间和日期输出。
相关推荐
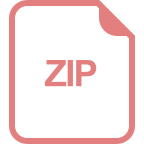
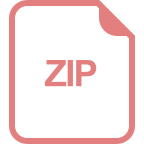
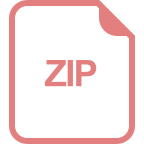















