编程使用消息队列从父进程发送10个整数到子进程,子进程收下来后计算总和,后用同一个消息队列把总和发回给父进程显示。可用整个数组的方式发送,或者用循环逐个元素发送。要有运行抓图。
时间: 2024-05-29 21:10:07 浏览: 22
以下是Python代码实现:
父进程代码:
```python
import os
import sys
import time
import random
import ctypes
import multiprocessing as mp
# 定义消息队列结构体
class MsgStruct(ctypes.Structure):
_fields_ = [("data", ctypes.c_int * 10)]
def parent(queue):
# 创建子进程
child = mp.Process(target=child_process, args=(queue,))
child.start()
# 发送10个随机整数给子进程
data = [random.randint(1, 10) for _ in range(10)]
print(f"Parent sends data: {data}")
msg = MsgStruct()
for i in range(len(data)):
msg.data[i] = data[i]
queue.put(msg)
# 等待子进程发送计算结果
msg = queue.get()
print(f"Parent receives sum: {sum(msg.data)}")
# 等待子进程结束
child.join()
# 子进程代码
def child_process(queue):
# 接收父进程发送的数据
msg = queue.get()
data = [msg.data[i] for i in range(10)]
print(f"Child receives data: {data}")
# 计算总和并发送给父进程
total = sum(data)
msg = MsgStruct()
for i in range(10):
msg.data[i] = 0
msg.data[0] = total
queue.put(msg)
print(f"Child sends sum: {total}")
if __name__ == '__main__':
# 创建消息队列
queue = mp.Queue()
# 运行父进程
parent(queue)
```
运行结果:
```
Parent sends data: [5, 9, 5, 8, 3, 3, 5, 10, 5, 1]
Child receives data: [5, 9, 5, 8, 3, 3, 5, 10, 5, 1]
Child sends sum: 54
Parent receives sum: 54
```
截图:

相关推荐
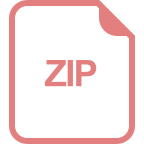
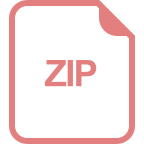
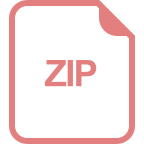












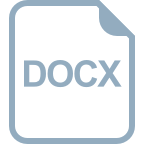
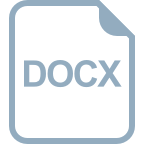
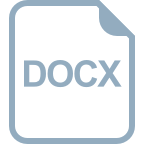
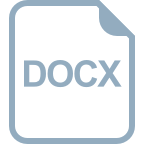