contours, hierarchy = cv2.findContours(binary, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
时间: 2023-08-13 15:26:47 浏览: 112
cv2.findContours()是OpenCV中的一个函数,用于在二进制图像中查找轮廓。该函数的语法如下:
contours, hierarchy = cv2.findContours(image, mode, method, offset)
其中,image是需要查找轮廓的二进制图像,mode是轮廓检索模式,method是轮廓近似方法,offset是可选参数,表示轮廓点坐标的偏移量。
在给定的二进制图像中,cv2.findContours()函数会找到所有的轮廓,并将每个轮廓表示为一组点的集合。这些点的集合存储在contours变量中。hierarchy变量包含关于轮廓层次结构的信息。
cv2.RETR_LIST表示提取所有轮廓,不建立轮廓间的层次关系。cv2.CHAIN_APPROX_SIMPLE表示轮廓近似方法,表示只保留轮廓的拐点信息,将轮廓形状用直线来逼近。
相关推荐
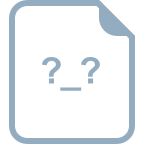
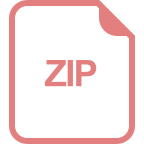
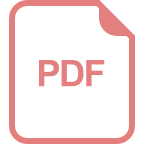
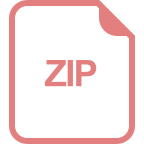
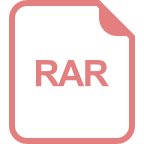
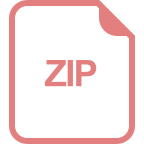
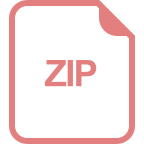