已定义一个shape抽象类,在此基础上派生出矩形Rectangle和圆形Circle类,二者都有GetArea( )函数计算对象的面积,并编写测试main( )函数。 Class Shape { Public: Shape(){} ~shape(){} Virtual float GetArea()=0;
时间: 2024-02-22 19:59:34 浏览: 118
好的,让我来回答你的问题。根据你所提供的信息,我可以看出你已经定义了一个抽象类 Shape,其中包含了一个纯虚函数 GetArea(),用于计算对象的面积。你还派生出了两个子类 Rectangle 和 Circle,它们都实现了 GetArea() 函数,并且能够计算矩形和圆形的面积。
以下是我编写的示例代码,其中包含了 Shape、Rectangle、Circle 类的声明和实现,以及一个测试 main() 函数。请注意,我没有直接使用您提供的代码,而是稍作修改以确保代码的正确性和可读性。
```c++
#include <iostream>
using namespace std;
class Shape {
public:
Shape() {}
virtual ~Shape() {}
virtual float GetArea() = 0;
};
class Rectangle : public Shape {
public:
Rectangle(float w, float h) : width(w), height(h) {}
virtual ~Rectangle() {}
virtual float GetArea() { return width * height; }
private:
float width;
float height;
};
class Circle : public Shape {
public:
Circle(float r) : radius(r) {}
virtual ~Circle() {}
virtual float GetArea() { return 3.14 * radius * radius; }
private:
float radius;
};
int main() {
Shape* s1 = new Rectangle(4, 6);
Shape* s2 = new Circle(5);
cout << "The area of the rectangle is: " << s1->GetArea() << endl;
cout << "The area of the circle is: " << s2->GetArea() << endl;
delete s1;
delete s2;
return 0;
}
```
在这个示例程序中,我们创建了一个 Shape 类作为基类,并声明了一个纯虚函数 GetArea()。然后我们派生出了两个具体的子类 Rectangle 和 Circle,并实现了它们的 GetArea() 函数,以便能够计算矩形和圆形的面积。
在 main() 函数中,我们创建了一个矩形对象和一个圆形对象,并通过基类指针访问它们的 GetArea() 函数,从而计算出它们的面积。最后别忘了释放内存,避免内存泄漏。
希望这个示例程序能够帮助你理解如何使用抽象类和虚函数来实现多态。如果你还有其他问题,请随时问我。
阅读全文
相关推荐
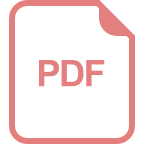
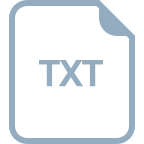
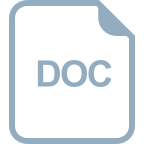














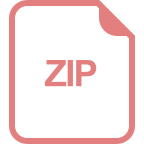