已定义一个shape抽象类,在此基础上派生出矩形Rectangle和圆形Circle类,二者都有GetArea( )函数计算对象的面积,并编写测试main( )函数。 Class Shape { Public: Shape(){} ~shape(){} Virtual float GetArea()=0; }
时间: 2024-02-19 21:57:52 浏览: 73
好的,根据你的要求,我写了如下的代码:
```c++
#include <iostream>
using namespace std;
class Shape {
public:
Shape() {}
virtual ~Shape() {}
virtual float GetArea() = 0;
};
class Rectangle : public Shape {
public:
Rectangle(float w, float h) : width(w), height(h) {}
~Rectangle() {}
float GetArea() {
return width * height;
}
private:
float width;
float height;
};
class Circle : public Shape {
public:
Circle(float r) : radius(r) {}
~Circle() {}
float GetArea() {
return 3.14159 * radius * radius;
}
private:
float radius;
};
int main() {
Shape* shapes[2];
shapes[0] = new Rectangle(2.0, 3.0);
shapes[1] = new Circle(1.0);
for (int i = 0; i < 2; i++) {
cout << "Shape " << i << " area: " << shapes[i]->GetArea() << endl;
delete shapes[i];
}
return 0;
}
```
在这个代码中,我们首先定义了一个抽象类 `Shape`,其中包含一个纯虚函数 `GetArea()`,表示计算对象面积的操作。然后我们派生出了两个类 `Rectangle` 和 `Circle`,分别表示矩形和圆形,并实现了它们的 `GetArea()` 函数。在 `main()` 函数中,我们创建了一个 `Shape` 类型的指针数组,并分别用 `Rectangle` 和 `Circle` 的对象来初始化其中的元素。然后我们遍历这个数组,输出每个对象的面积,并释放掉动态分配的内存。
你可以将这个代码保存为一个名为 `shape.cpp` 的文件,然后在命令行中使用如下命令进行编译和运行:
```
g++ shape.cpp -o shape
./shape
```
然后你就可以看到输出结果了。
阅读全文
相关推荐
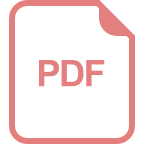
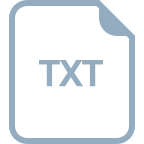
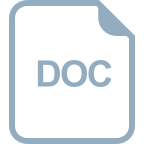














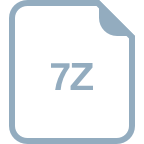
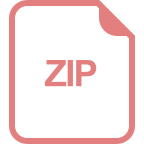
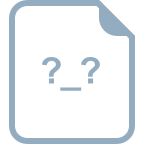