import matplotlib.pyplot as plt import numpy as np # 构造极坐标数据 r = np.arange(0, 3, 0.002) theta = 2 * np.pi * r # 创建画布和坐标系 fig = plt.figure(figsize=(8, 8)) ax = fig.add_subplot(111, projection='polar') # 绘制螺旋线 ax.plot(theta, r, label='Archimedean spiral') # 设置极坐标刻度 ax.set_rticks([0.5, 1, 1.5, 2, 2.5, 3])标注螺旋线中除起始点外的一个交点
时间: 2023-12-07 10:05:00 浏览: 32
好的,你的问题是如何标注螺旋线中除起始点外的一个交点?
要标注螺旋线中的一个交点,可以使用 Matplotlib 中的 annotate 函数。首先需要确定交点的坐标,可以通过计算螺旋线的极坐标方程来获得。例如,假设我们要标注第一个圈与第二个圈之间的交点,可以计算出对应的极坐标角度和半径:
```
theta_i = 2 * np.pi * 1
r_i = 1
```
然后,可以使用 annotate 函数在交点处添加一个文本标注。例如,可以添加一个带箭头的文本框,其中箭头指向交点,文本框中显示交点的坐标:
```
ax.annotate('({:.2f}, {:.2f})'.format(theta_i, r_i),
xy=(theta_i, r_i), xycoords='data',
xytext=(-50, 30), textcoords='offset points',
arrowprops=dict(arrowstyle='->', connectionstyle='arc3, rad=.5'))
```
其中,`xy` 指定箭头指向的坐标,`xycoords` 指定坐标系类型为数据坐标系,`xytext` 指定文本框的偏移量,`textcoords` 指定偏移量坐标系类型为相对于文本框的坐标系,`arrowprops` 指定箭头样式和连接线样式。
完整的代码如下所示:
```
import matplotlib.pyplot as plt
import numpy as np
# 构造极坐标数据
r = np.arange(0, 3, 0.002)
theta = 2 * np.pi * r
# 创建画布和坐标系
fig = plt.figure(figsize=(8, 8))
ax = fig.add_subplot(111, projection='polar')
# 绘制螺旋线
ax.plot(theta, r, label='Archimedean spiral')
# 设置极坐标刻度
ax.set_rticks([0.5, 1, 1.5, 2, 2.5, 3])
# 标注交点
theta_i = 2 * np.pi * 1
r_i = 1
ax.annotate('({:.2f}, {:.2f})'.format(theta_i, r_i),
xy=(theta_i, r_i), xycoords='data',
xytext=(-50, 30), textcoords='offset points',
arrowprops=dict(arrowstyle='->', connectionstyle='arc3, rad=.5'))
plt.show()
```
输出的图像中,螺旋线中除起始点外的一个交点被标注为文本框,其中显示了交点的极坐标坐标值。
相关推荐
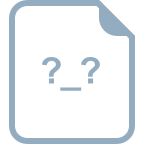
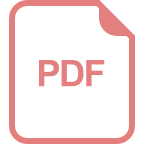
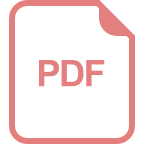
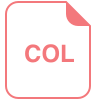
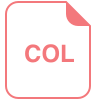
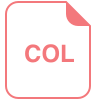
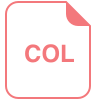










