c语言 创建一个二叉树并实现先序递归,中序递归,后序递归,先序非递归,中序非递归和后序非递归的遍历,其中用到了自己创建的栈的mystack.h头文件
时间: 2024-10-25 14:18:52 浏览: 42
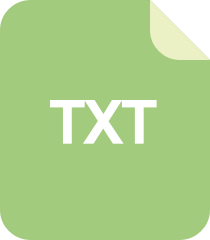
二叉树建立及遍历(非递归 C语言)

在C语言中,二叉树是一种常见的数据结构,用于组织元素并支持高效的查找、插入和删除操作。首先,我们定义一个二叉树节点的基本结构:
```c
#include "mystack.h" // 假设mystack.h头文件已包含栈的定义
typedef struct TreeNode {
int data;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 创建一个空的二叉树
TreeNode* createTree() {
return (TreeNode*)malloc(sizeof(TreeNode));
}
// 先序遍历(根-左-右)
void preorderRecursion(TreeNode* node) {
if (node != NULL) {
printf("%d ", node->data);
preorderRecursion(node->left);
preorderRecursion(node->right);
}
}
// 中序遍历(左-根-右)
void inorderRecursion(TreeNode* node) {
if (node != NULL) {
inorderRecursion(node->left);
printf("%d ", node->data);
inorderRecursion(node->right);
}
}
// 后序遍历(左-右-根)
void postorderRecursion(TreeNode* node) {
if (node != NULL) {
postorderRecursion(node->left);
postorderRecursion(node->right);
printf("%d ", node->data);
}
}
// 非递归先序遍历(利用栈)
void preorderNonRecursive(TreeNode* root) {
if (root == NULL) return;
stack_push(mystack, root);
while (!stack_is_empty(mystack)) {
TreeNode* temp = stack_pop(mystack);
printf("%d ", temp->data);
if (temp->right) stack_push(mystack, temp->right);
if (temp->left) stack_push(mystack, temp->left);
}
}
// 类似的,你可以为中序和后序遍历也编写非递归版本,只是顺序会有所不同,即先压入右子节点再压入左子节点。
阅读全文
相关推荐
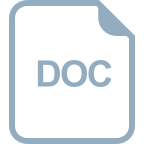
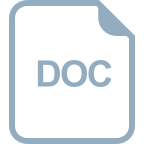


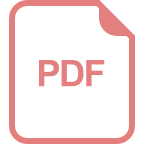
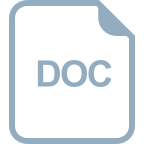
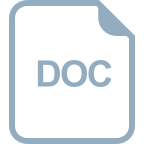









